By: Team T09-4
Since: Aug 2018
Licence: MIT
- 1. Setting up
- 2. Design
- 3. Implementation
- 3.1. Undo/Redo feature
- 3.2. Auto Complete Feature
- 3.3. List history feature
- 3.4. Sort records feature
- 3.5. View summary feature
- 3.6. View category breakdown feature
- 3.7. View current month’s breakdown
- 3.8. Find records by tag feature
- 3.9. Limit features
- 3.10. Delete all the record whose date is required
- 3.11. Export the records from Savee to the Excel file.
- 3.12. Import the record data from the Excel file to Savee
- 3.13. Archive the record data from Savee to the Excel file
- 3.14. Draw a line chart automatically inside the Excel sheet
- 3.15. [Proposed] Data Encryption
- 3.16. Logging
- 3.17. Configuration
- 4. Documentation
- 5. Testing
- 6. Dev Ops
- Appendix A: Suggested Programming Tasks to Get Started
- Appendix B: Product Scope
- Appendix C: User Stories
- Appendix D: Use Cases
- Appendix E: Non Functional Requirements
- Appendix F: Glossary
- Appendix G: Product Survey
- Appendix H: Instructions for Manual Testing
- H.1. Launch and Shutdown
- H.2. Listing by date
- H.3. Sorting records
- H.4. Finding Records by Tag
- H.5. Viewing summary
- H.6. Viewing statistics about category breakdown
- H.7. Viewing current month’s breakdown
- H.8. Adding a normal limit
- H.9. Editing an existing normal limit
- H.10. Deleting an existing normal limit
- H.11. Adding a monthly limit
- H.12. Deleting the monthly limit
- H.13. Checking all the limits
- H.14. Deleting all records whose date is required.
- H.15. Archiving records data from Savee to Excel file and store it in a specific directory.
- H.16. Import all record data from Excel file to Savee
- H.17. Exporting records data from Savee to Excel file and store it in a specific directory.
- H.18. Auto Complete Feature
- H.19. Saving data
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
seedu.planner.MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the SE-EDU branding and refer to the se-edu/addressbook-level4
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to se-edu/addressbook-level4
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at Appendix A, Suggested Programming Tasks to Get Started.
2. Design
2.1. Architecture
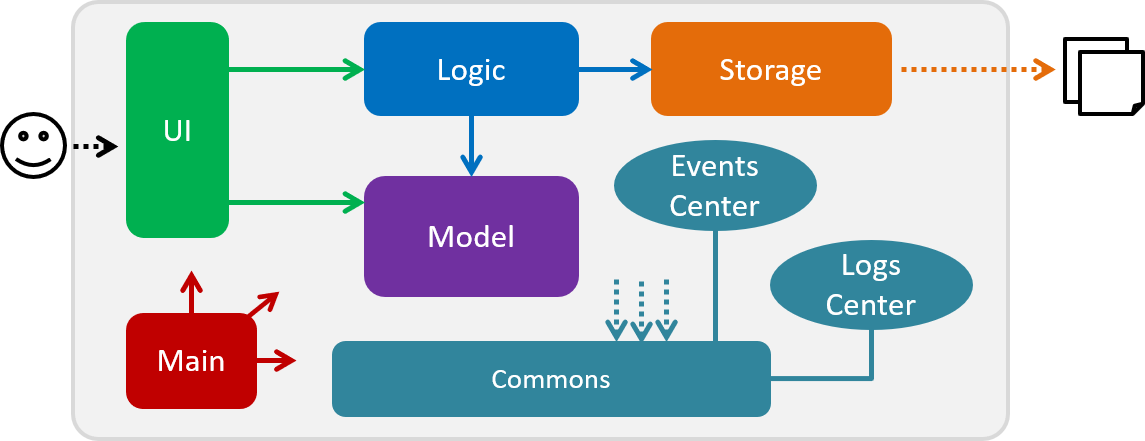
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
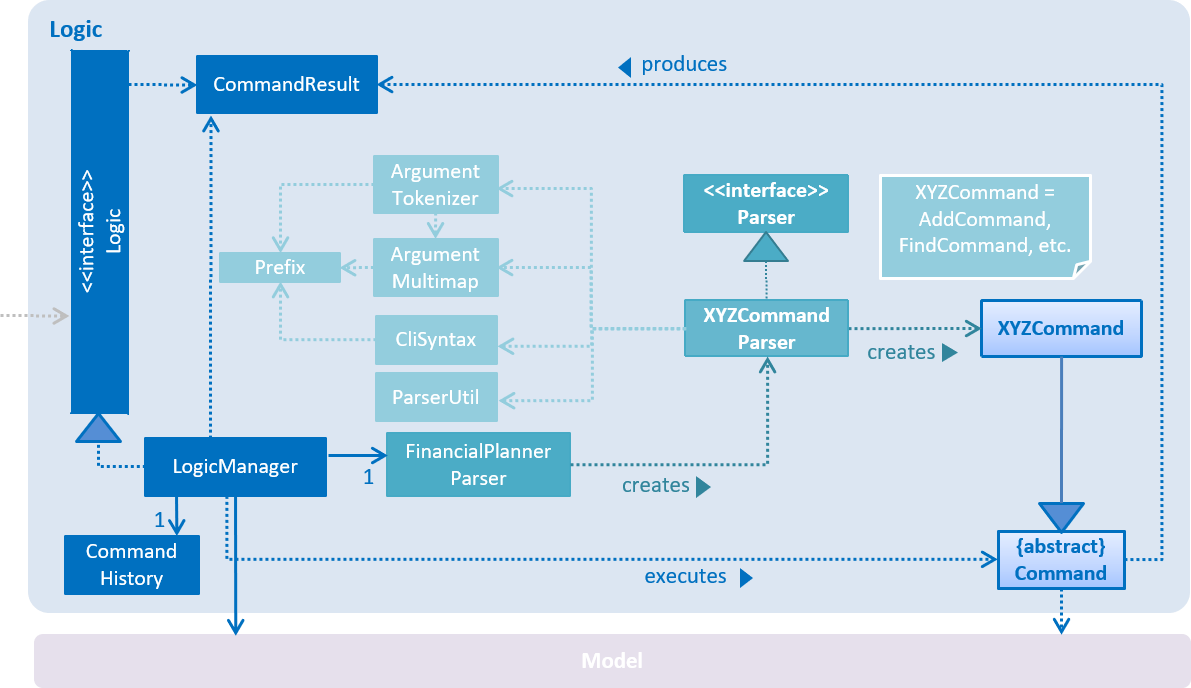
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
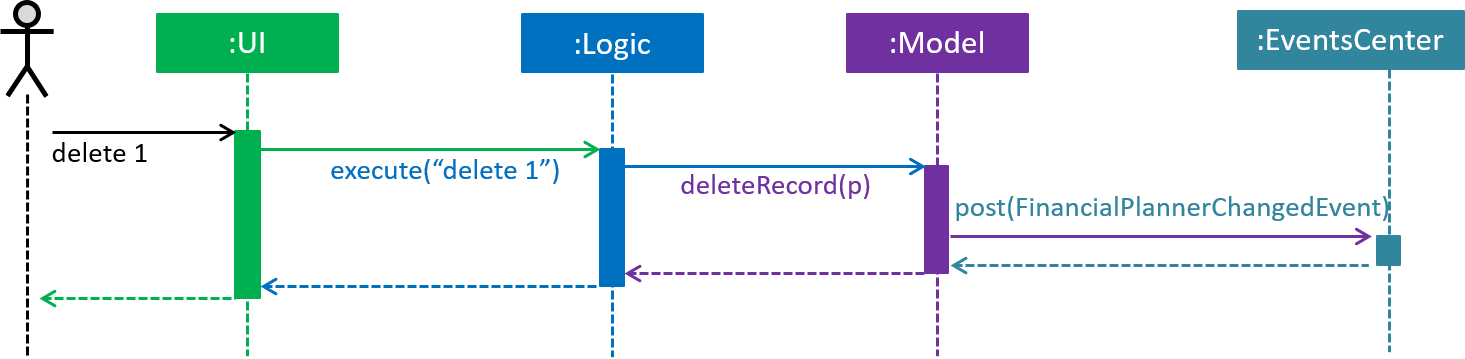
delete 1
command (part 1)
Note how the Model simply raises a FinancialPlannerChangedEvent when the Financial Planner data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
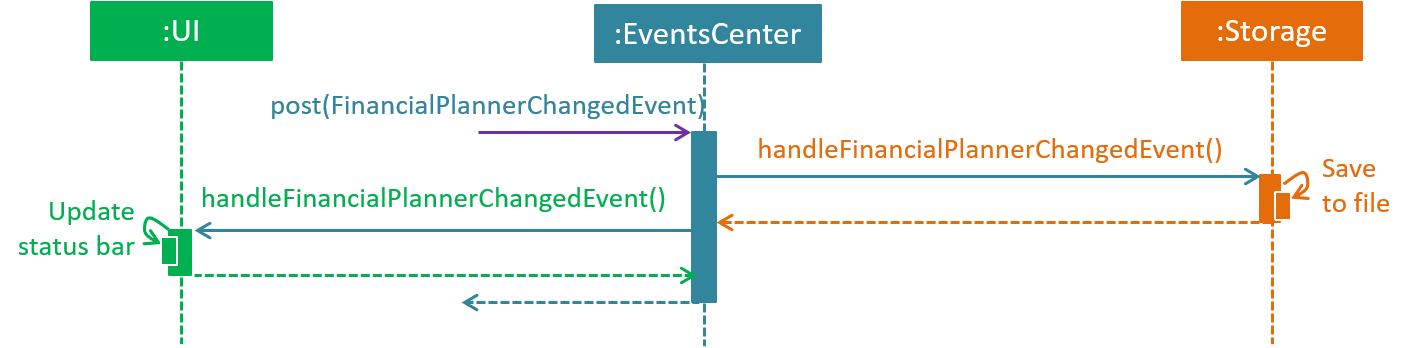
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
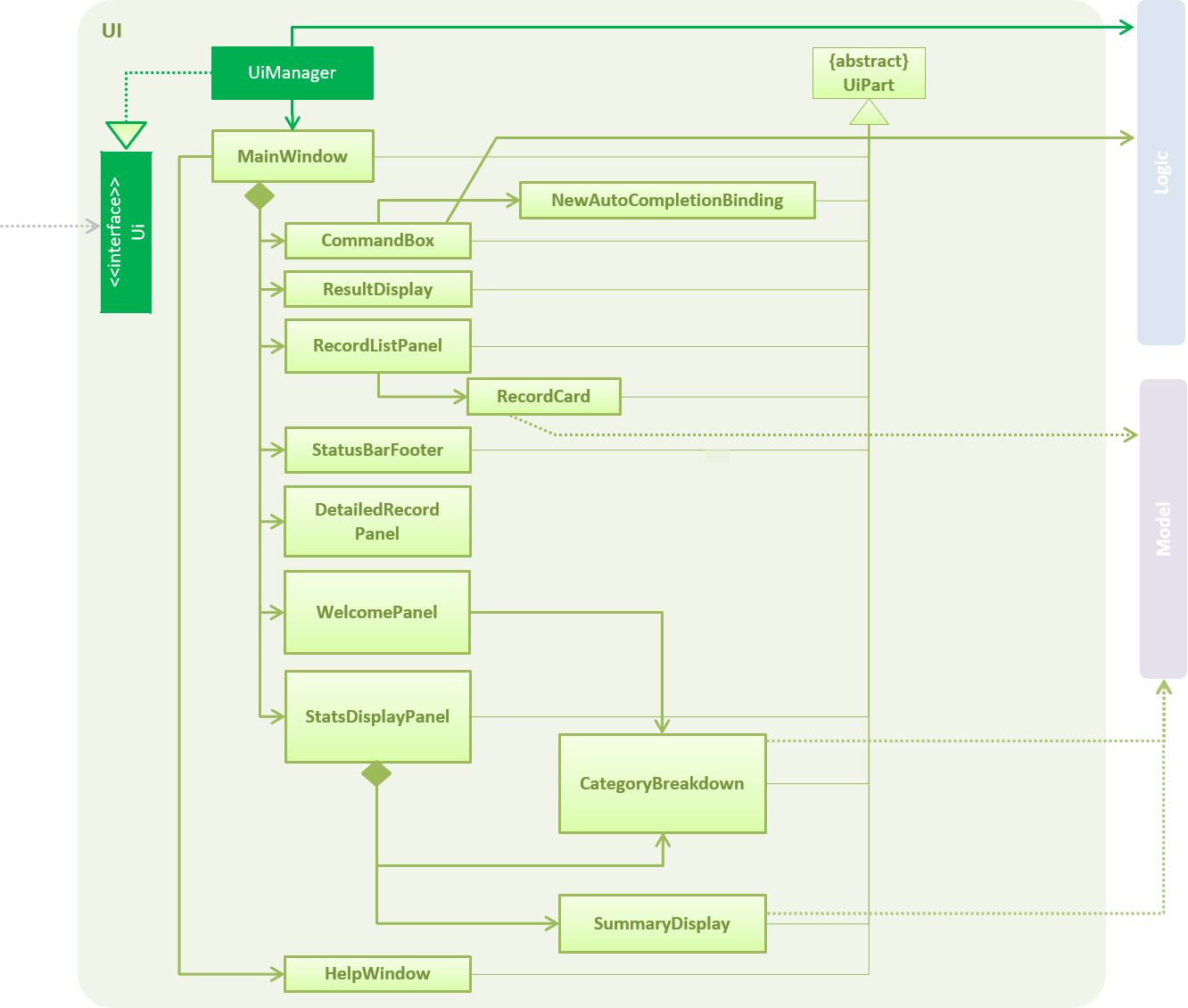
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
, WelcomePanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder.
For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
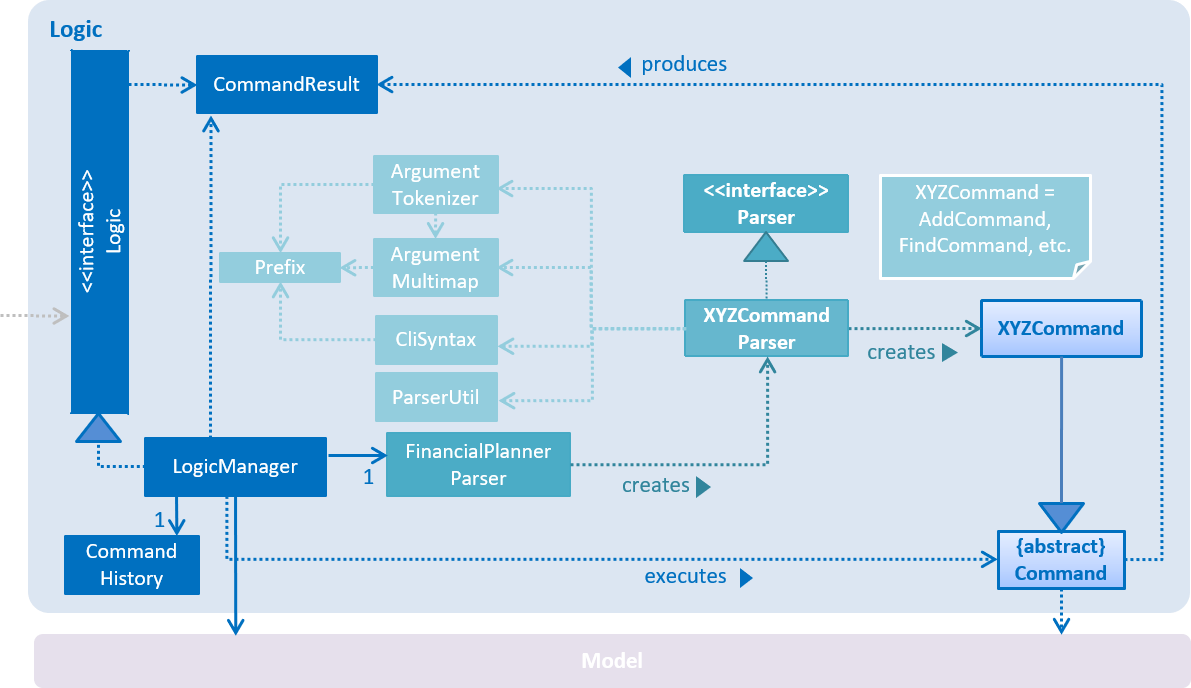
API :
Logic.java
-
Logic
uses theFinancialPlannerParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a record) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
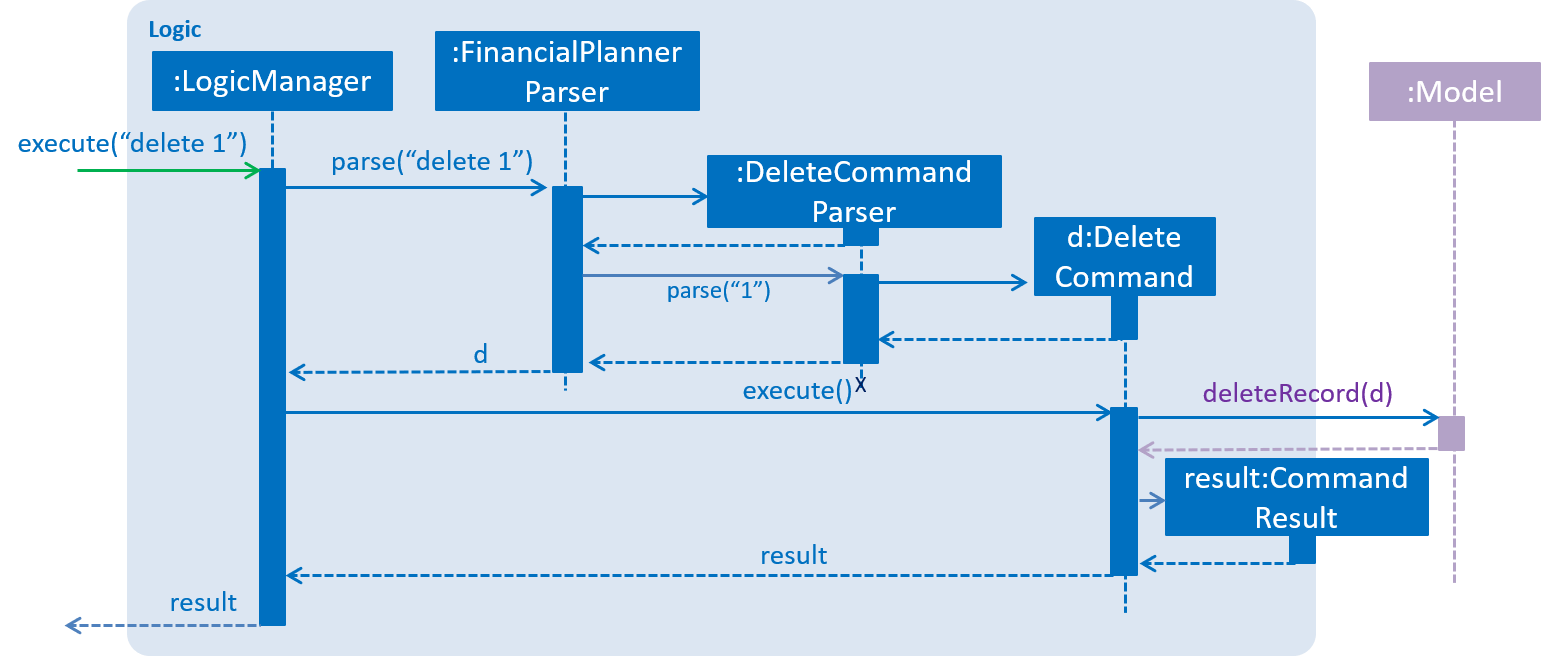
delete 1
Command2.4. Model component
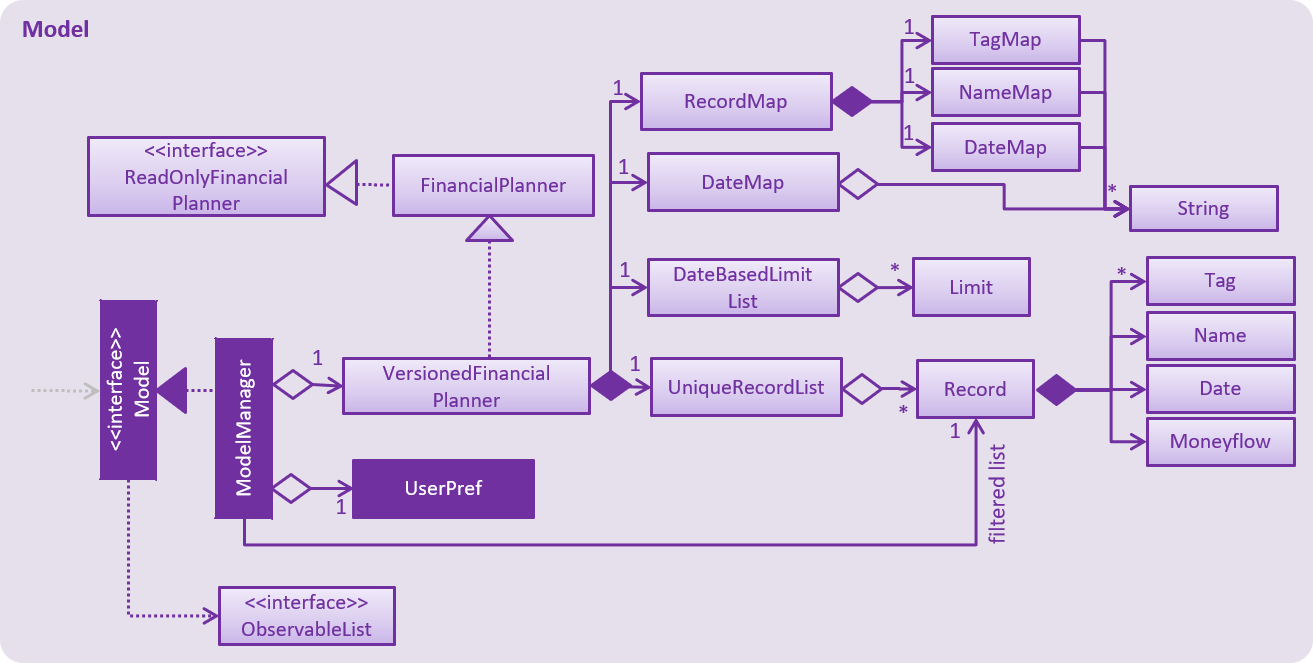
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Financial Planner data.
-
exposes an unmodifiable
ObservableList<Record>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
exposes an unmodifiable
ObservableList<Limit>
that can beobserved
-
contains a
FilteredList<Record>
of all records in the current month which is updated automatically and has listeners bound to it that will notify the Ui of any changes -
does not depend on any of the other three components.
As a more OOP model, we can store a Tag list in Financial Planner , which Record can reference. This would allow Financial Planner to only require one Tag object per unique Tag , instead of each Person needing their own Tag object. An example of how such a model may look like is given below.![]() |
2.5. Storage component
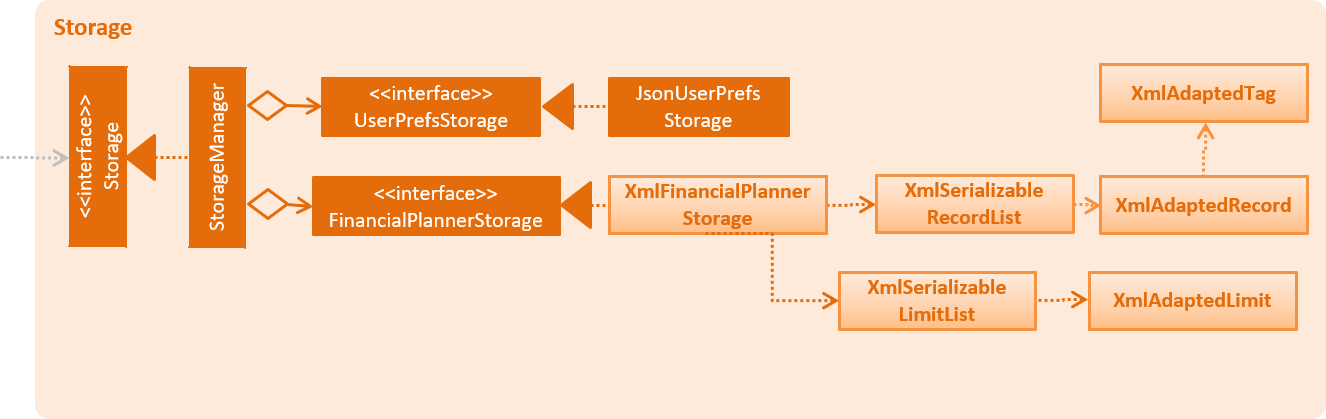
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the RecordList data in xml format and read it back.
-
can save the LimitList data in xml format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.planner.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Undo/Redo feature
3.1.1. Current Implementation
The undo/redo mechanism is facilitated by VersionedFinancialPlanner
.
It extends FinancialPlanner
with an undo/redo history, stored internally as an financialPlannerStateList
and
currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedFinancialPlanner#commit()
— Saves the current financial planner state in its history. -
VersionedFinancialPlanner#undo()
— Restores the previous financial planner state from its history. -
VersionedFinancialPlanner#redo()
— Restores a previously undone financial planner state from its history.
These operations are exposed in the Model
interface as Model#commitFinancialPlanner()
,
Model#undoFinancialPlanner()
and Model#redoFinancialPlanner()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedFinancialPlanner
will be initialized with
the initial financial planner state, and the currentStatePointer
pointing to that single financial planner state.

Step 2. The user executes delete 5
command to delete the 5th person in the financial planner. The delete
command calls
Model#commitFinancialPlanner()
, causing the modified state of the financial planner after the delete 5
command executes
to be saved in the financialPlannerStateList
, and the currentStatePointer
is shifted to the newly inserted financial planner state.
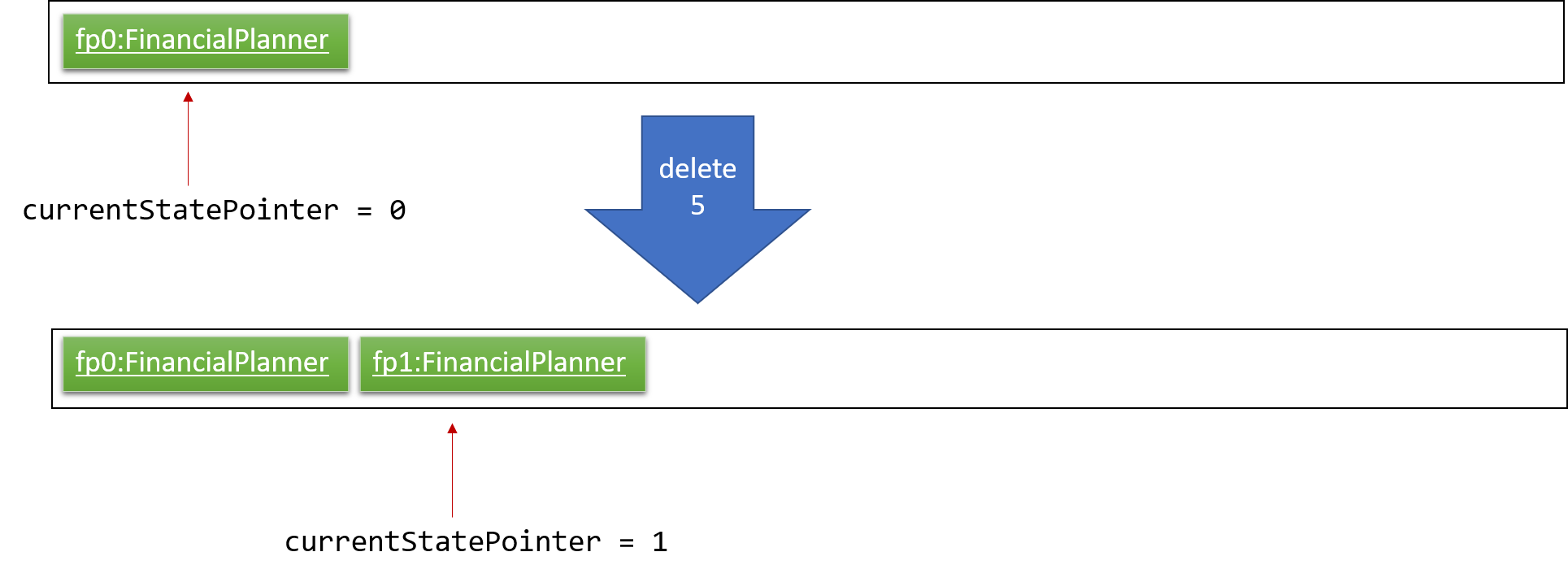
Step 3. The user executes add n/Mala …
to add a new record. The add
command also calls
Model#commitFinancialPlanner()
, causing another modified financial planner state to be saved into the
financialPlannerStateList
.
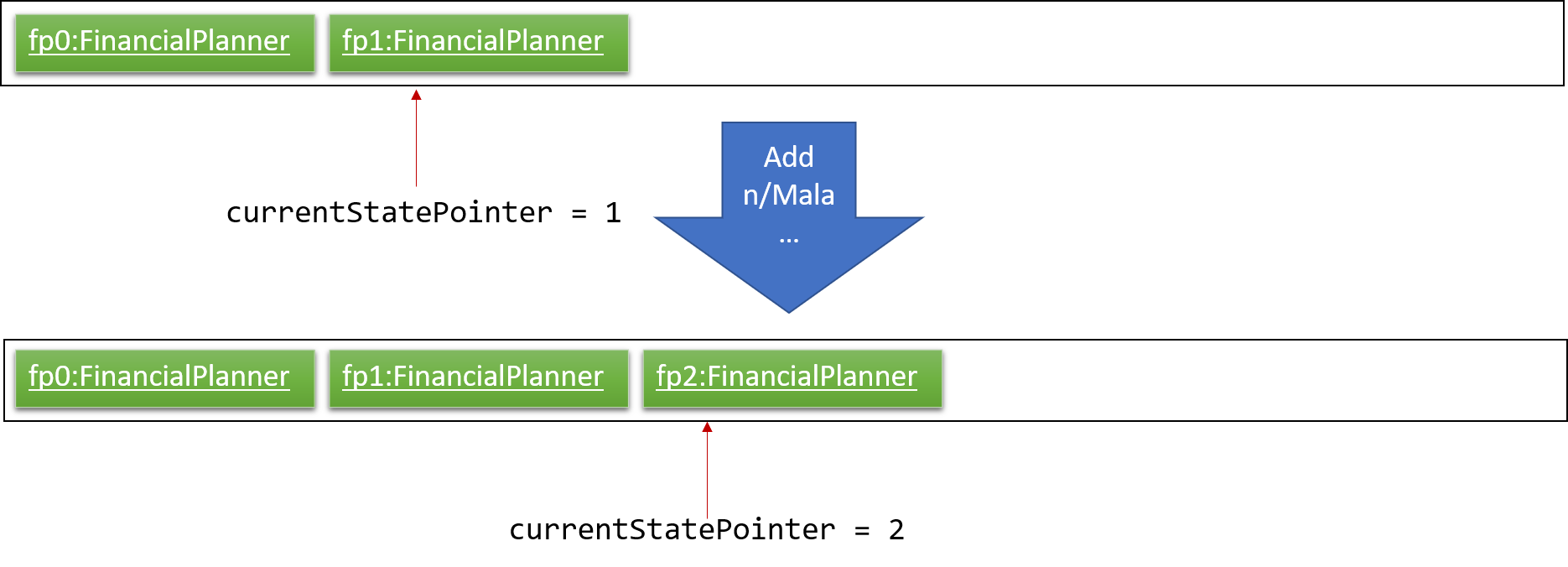
If a command fails its execution, it will not call Model#commitFinancialPlanner() , so the financial planner state will
not be saved into the financialPlannerStateList .
|
Step 4. The user now decides that adding the record was a mistake, and decides to undo that action by executing the
undo
command. The undo
command will call Model#undoFinancialPlanner()
, which will shift the currentStatePointer
once to the left, pointing it to the previous financial planner state, and restores the financial planner to that state.
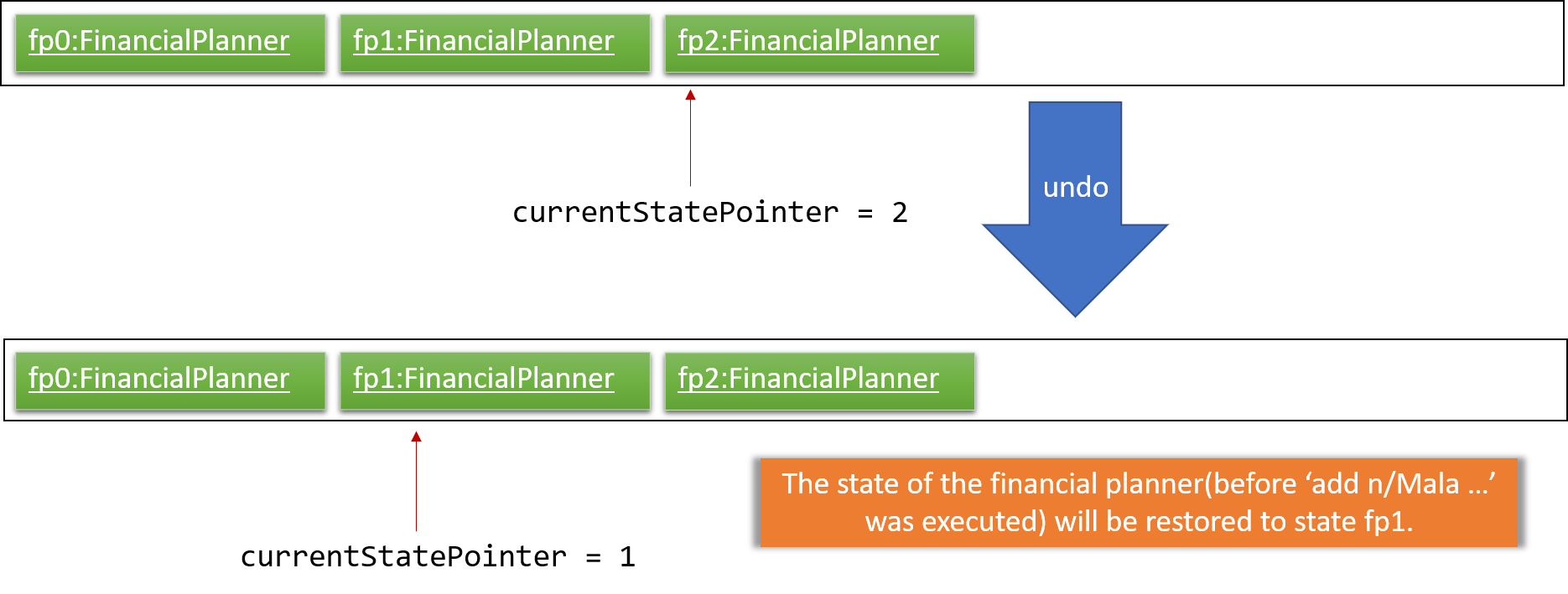
If the currentStatePointer is at index 0, pointing to the initial financial planner state, then there are no previous
financial planner states to restore. The undo command uses Model#canUndoFinancialPlanner() to check if this is the case.
If so, it will return an error to the user rather than attempting to perform the undo.
|
The following sequence diagram shows how the undo operation works:
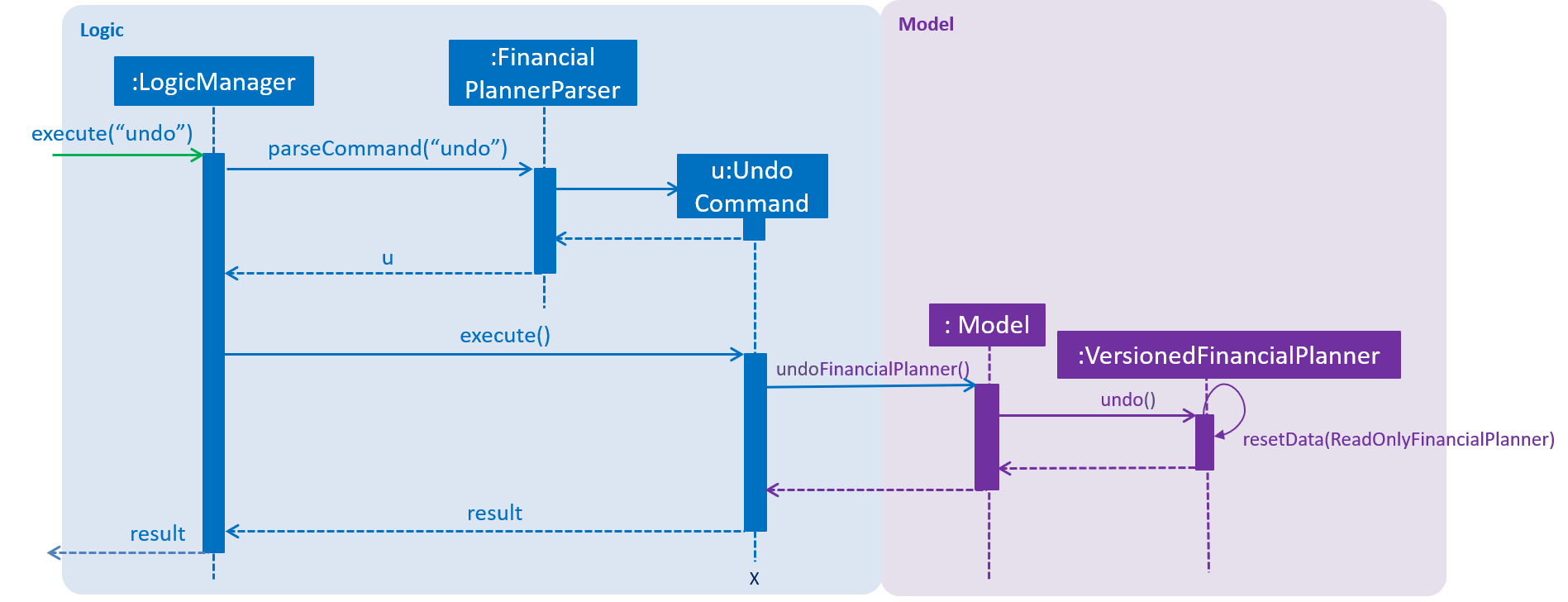
The redo
command does the opposite — it calls Model#redoFinancialPlanner()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the financial planner to that state.
If the currentStatePointer is at index financialPlannerStateList.size() - 1 , pointing to the latest financial planner
state, then there are no undone financial planner states to restore. The redo command uses Model#canRedoFinancialPlanner()
to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
|
Step 5. The user then decides to execute the command list
. Commands that do not modify the financial planner, such as
list
, will usually not call Model#commitFinancialPlanner()
, Model#undoFinancialPlanner()
or
Model#redoFinancialPlanner()
. Thus, the financialPlannerStateList
remains unchanged.
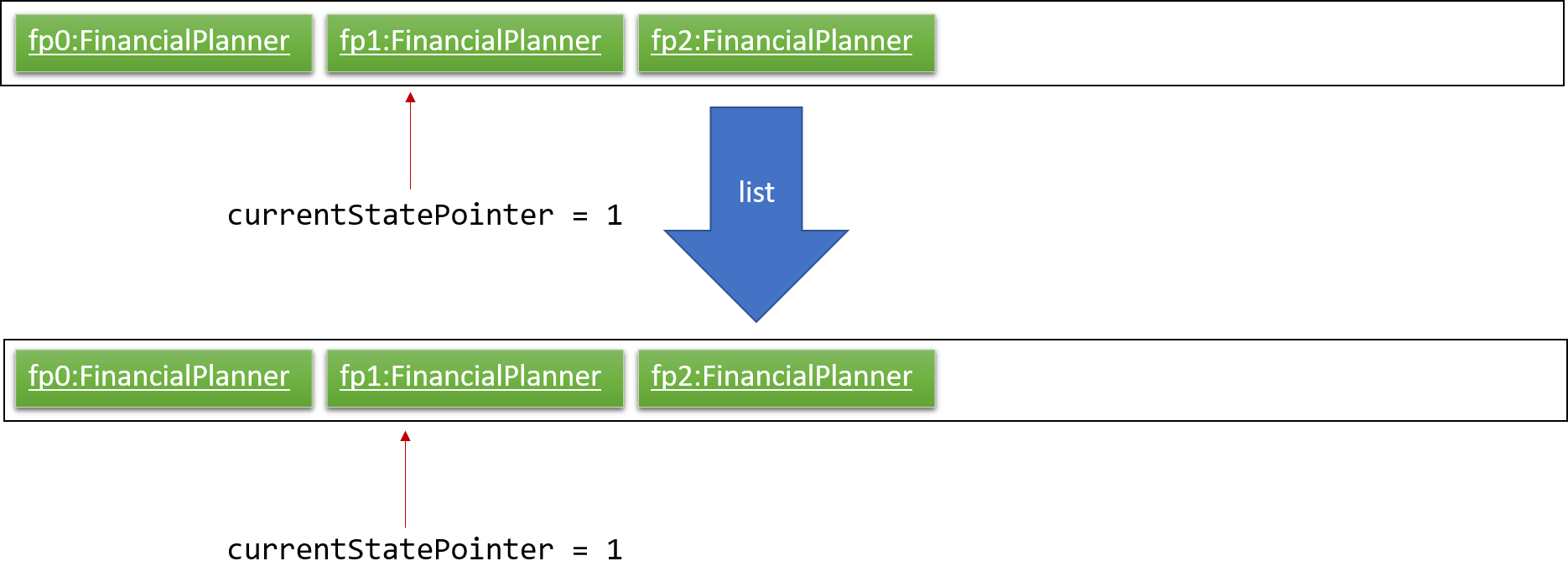
Step 6. The user executes clear
, which calls Model#commitFinancialPlanner()
. Since the currentStatePointer
is not pointing at the end of the financialPlannerStateList
, all financial planner states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the add n/David …
command. This is the behavior that most modern desktop applications follow.
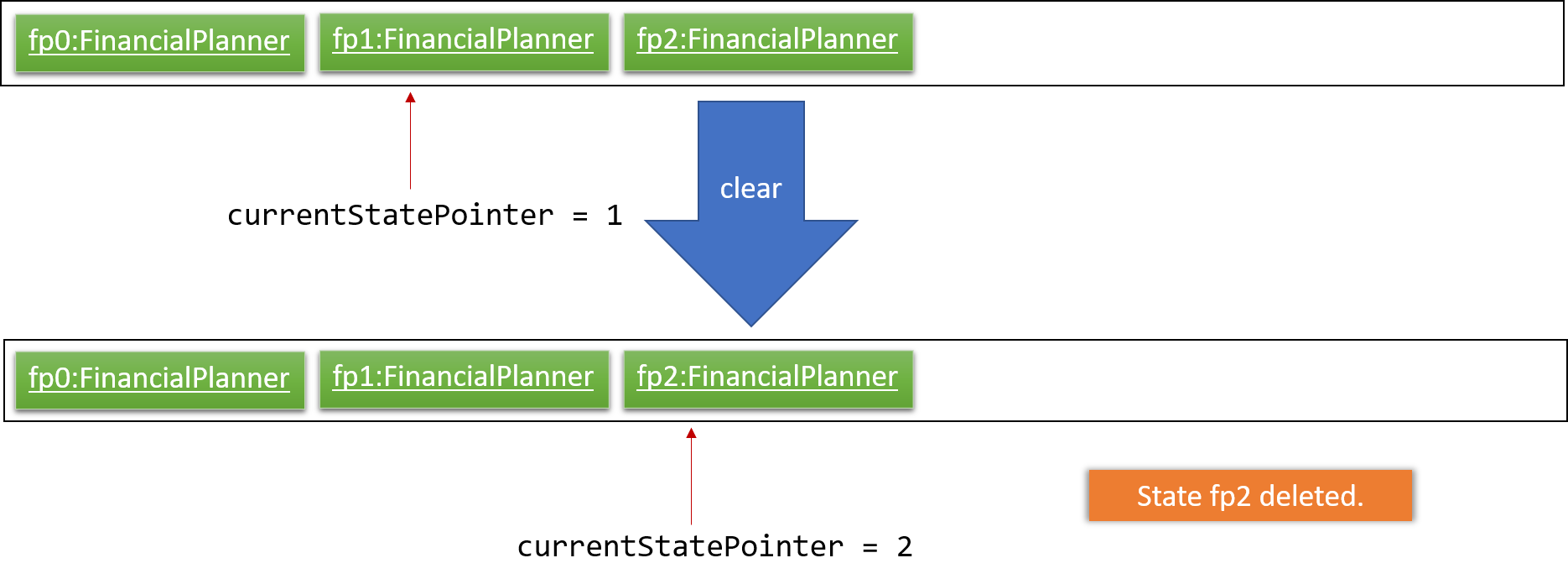
The following activity diagram summarizes what happens when a user executes a new command:
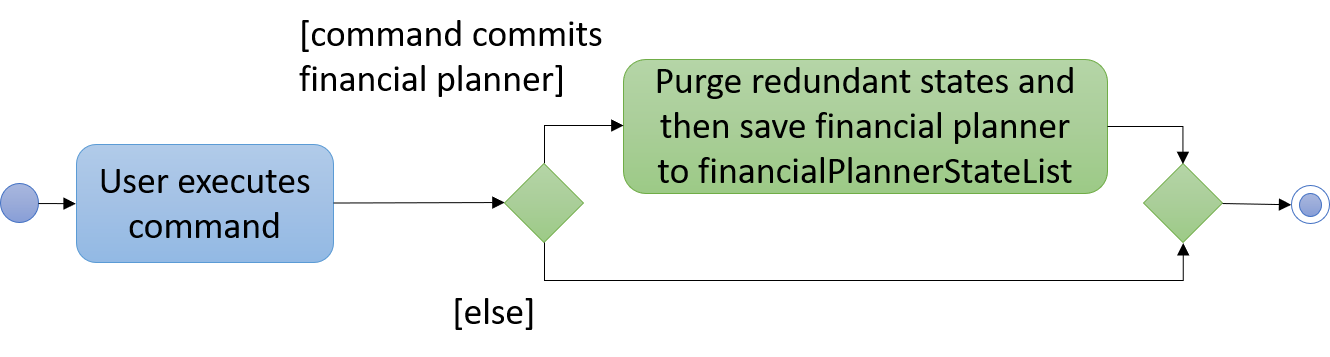
3.1.2. Design Considerations
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire financial planner.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the person being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use a list to store the history of financial planner states.
-
Pros: Easy for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andVersionedFinancialPlanner
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
3.2. Auto Complete Feature
3.2.1. Current Implementation
The autocomplete mechanism is facilitated by the NewAutoCompletionBinding
class. It represents the binding of the
autocomplete window to the CommandBox
and is the component that manages the interactions between the text input and
the contents of the autocomplete list that is displayed in the window. This feature observes the text input in the
CommandBox and displays a list of words in another window under the
CommandBox for the user to select and complete his current focus text - the word he is currently typing.
The key components in this class are the autoCompletionPopup
and the caretChangedListener
that perform the key
functionalities.
caretChangedListener
is an observer that checks the caret in the command box and is triggered whenever the caret
changes its position, whether a character is typed or the caret is moved using the arrow keys. When triggered, it reads
the text in the command box and checks the position of the caret, then extracts the current word of target to be
completed as well as the current input text and the prefix of the current word if any. Extraction is done by tracing back from the caret
position and as well as tracing forward. Any characters that are not a whitespace and are not separated by a whitespace
are considered to be the same word as the current word to be completed. The text before and after the word to completed
are also saved so they will not be lost upon completion.
This listener passes the extracted String input to the CustomSuggestionProvider
through updateSuggestions
.
CustomSuggestionProvider
reads the word to be competed and the input text. It trims and splits the input text and
find the index of the word to completed. It reads the first input first as it is the key command word and performs a
different operation on the input string depending on the command word as well as any possible following parameters.
Functions which are supported by autocomplete will parse the input individually through the different private methods
available in CustomSuggestionProvider
. Once it has decided which set of texts to be the new word suggestion bank,
it calls the CustomSuggestionProvider#updateSuggestions
overloaded method to update the SuggestionProvider
.
After updating the SuggestionProvider
, NewAutoCompletionBinding#SetUserInput
calls onUserInputChanged
which
is responsible for updating the suggestions list according to what the word to be completed is. As this class was
tweaked from the library implementation, the threading involved in this method will not be detailed. It updates
the list of suggestions
by comparing the user input word to the list of suggestions and passes the resultant list to the autoCompletionPopup
which will proceed to display the list of text in the popup below the command box.
Now back to the autoCompletionPopup
, whenever the user highlights a selected word input and selects it, the
autoCompletionPopup#setOnSuggestion
is triggered and will try to complete the word with the selected text and once
completed, it hides the popup and sets the caret to the end of the newly completed word.
The following sequence diagram shows how the auto completion operation works:
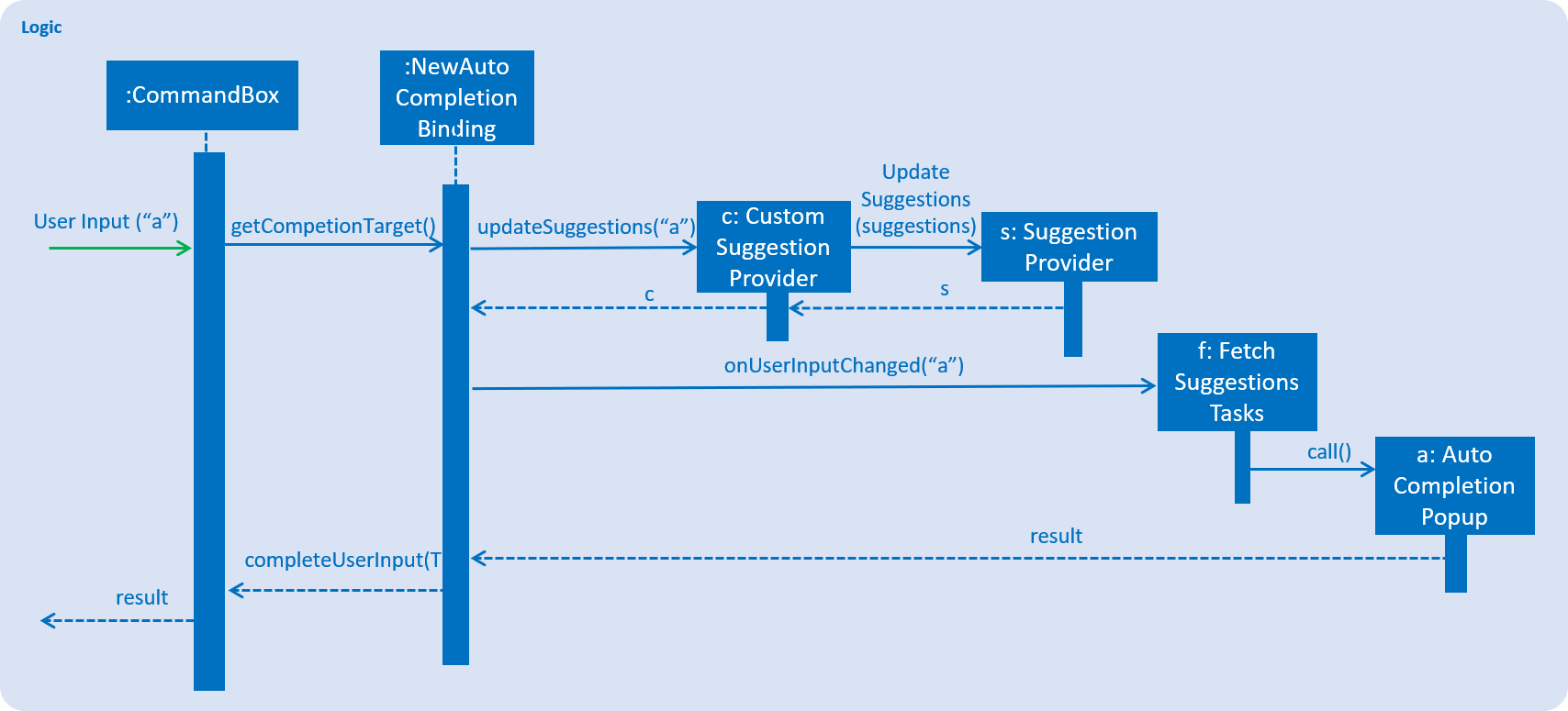
3.2.2. Design Considerations
Aspect: Method to Update Suggestion Bank to use for comparison to input word
-
Alternative 1 (current choice): Use listeners to the caret in the command box
-
Pros: Accurate parsing of input and able to detect which word is currently in focus.
-
Cons: Difficult to correctly parse text, as need to avoid whitespaces. Also led to many index array exceptions that can occur when checking the input.
-
-
Alternative 2: Use text listeners in the command box
-
Pros: Simple to implement, similar to library implementation. Safe and minimal room for errors.
-
Cons: Cannot autocomplete by word, can only complete the last word input in the command box. Unable to perform completion for texts before the last word.
-
Aspect: Method to compare input word to Suggestion Bank to obtain displayed list of words
-
Alternative 1 (current choice): Check if suggestion bank word begins with input word
-
Pros: Simple, straightforward autocomplete for users. Users that are already good at typing and spelling will have a breeze using this implementation.
-
Cons: Smaller range of completion texts as users need to accurately type the first few letters before getting a an expected completion suggestion.
-
-
Alternative 2: Check if suggestion bank word contains input word e.g type
tag
→ suggestsfindtag
-
Pros: User can type whichever word is convenient to them to obtain a suggestion they desire. Users who may not know the full spelling of a word and only partially will enjoy this.
-
Cons: Range of texts displayed may be excessive and not appealing to all users.
-
3.3. List history feature
3.3.1. Current Implementation
The list mechanism is facilitated by ModelManager
.
It represents an in-memory model of the FinancialPlanner and is the component which manages the interactions between the commands and the VersionedFinancialPlanner
.
ListCommand calls ModelManager#updateFilteredRecords
and passes in different predicates depending on the argument mode.
This feature has only one keyword list
but implements 3 different argument modes to allow users to access multiple versions of the same command.
The three argument modes are as listed below:
-
No Argument mode — Requires no arguments and returns the entire list of records in the FinancialPlanner.
-
Single Argument mode — Requires a single date and returns all records containing that date
-
Dual Argument mode — Requires 2 dates, a
start date
and anend date
. It returns all records containing dates within the time frame of start date and end date, inclusive of both start date and end date The mechanism that facilitates these modes can be found in theListCommandParser#parse
. Below is a overview of the mechanism:-
The input given by the user is passed to
ArgumentTokeniser#tokenise
to split the input separated by prefixes which returns aArgumentMultiMap
which contains a map with prefixes as keys and their associated input arguments as the value. -
The string associated with
d/
is then passed intoListCommandParser#splitByWhitespace
for further processing and returns an array. -
The argument mode is determined by the size of this array and the elements are further processed into
Date
objects, before creating and returning aListCommand
object.
-
The ListCommand
has two constructors which makes use of overloading to reduce code complexity, one with no argument and the other one with 2 Date
arguments.
The following sequence diagram shows how the list operation works:
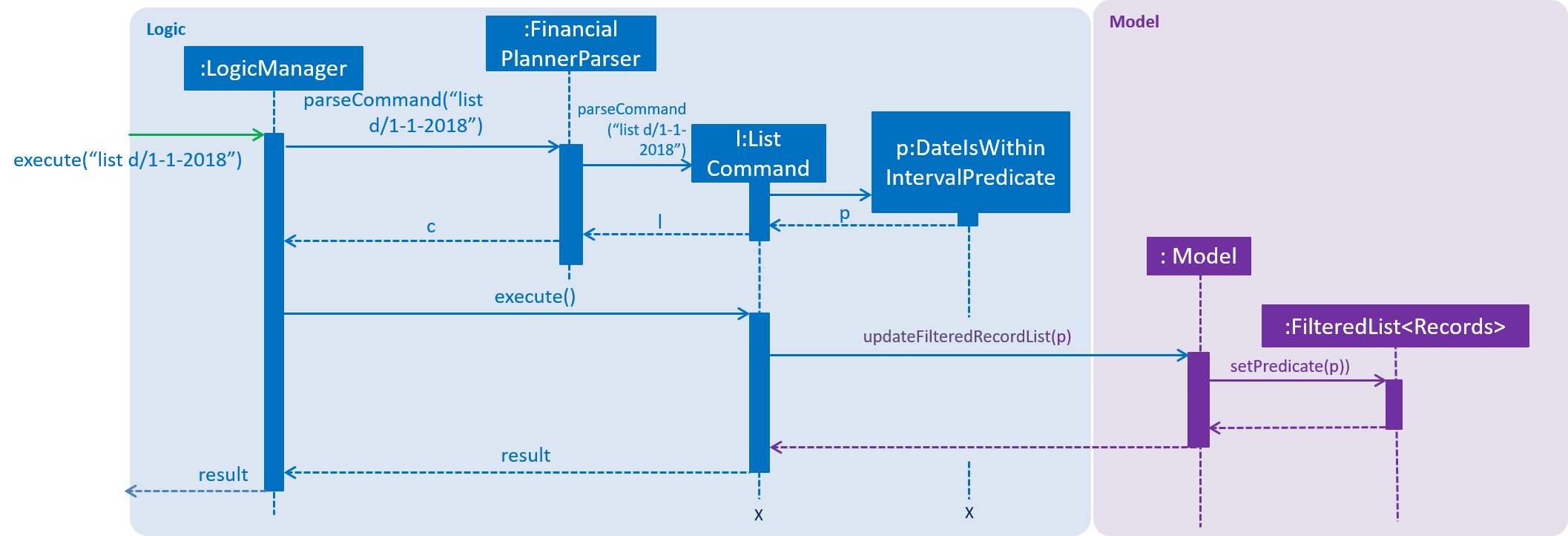
For simplicity, interactions with the UI is not shown in the diagram above.
The update of the UI RecordListPanel
is done through the event system. FilteredList
is a type of ObservableList
implemented by the Java 8 API and it will propagate any changes to the list to any listeners listening to it. This
listener is present in RecordListPanel
and will update the UI list automatically.
3.3.2. Design Considerations
Aspect: Data structure to support listing of records
-
Alternative 1 (current choice): Uses a FilteredList that is tracked by the UI. FilteredLIst is a wrapper around the ObservableList<Record> that is stored in UniquePersonList which allows for any changes in the observable list to be propagated to the filtered list automatically.
-
Pros: Easy to implement
-
Cons: May take a significantly longer time to list records if there are many records spanning across a large timeframe.
-
-
Alternative 2: Implement a HashMap with Date as the key and Record as the value.
-
Pros: Allows for constant time complexity to access any elements. Hence, listing records can potentially be faster.
-
Cons: Current UI implementation relies on
FilteredList
. In order for UI to be compatible with the new data structure, the UI may need to change its implementation toObservableMap
instead. Alternatively, one can utilise aHashMap
to first generate the list and pass the list reference intoFilteredList
. However, there is a need to code a filter function.
-
3.4. Sort records feature
3.4.1. Current Implementation
The sort mechanism is facilitated by ModelManager
. It extends FinancialPlanner
with a component that sorts the
internal list of records. SortCommand calls ModelManager#sortFilteredRecordList
and passes in the category to be
sorted by and the sort order.
This feature has one keyword sort
and takes in arguments of either category or order of sort. Keywords are not
case sensitive.
Category can be either of the following keywords: name
, date
, money
, moneyflow
Order can be either of the following keywords: desc
- descending, asc
- ascending
This feature has 2 different kind of modes as follows:
-
Single Argument Mode - Input argument can be either the category or the order of sort
-
If category specified, records are sorted in ascending order of that category
-
If order specified, records will be sorted by name in the specified order
-
-
Duo Argument Mode - Input arguments must contain only 1 category and only 1 order, and can be input in no particular order
The input given by the user is passed to SortCommandParser
to split the input separated by whitespaces to ensure
there is either only one or two arguments input by the user. These arguments are stored in an array of strings and
the size of the array determines the mode of the command.
The strings are compared to two sets of strings containing the supported categories and orders of the function.
The string of the category and a boolean representing whether the records are to be reversed will then be passed to
ModelManager
to sort the records.
Since the displayed list in the UI is a FilteredList
which is a wrapper for the underlying list UniqueRecordList
structure,
sorting the internal list of records in versionedFinancialPlanner
will post an event that notifies the UI to update
the displayed list.
The following sequence diagram shows how the sort operation works:
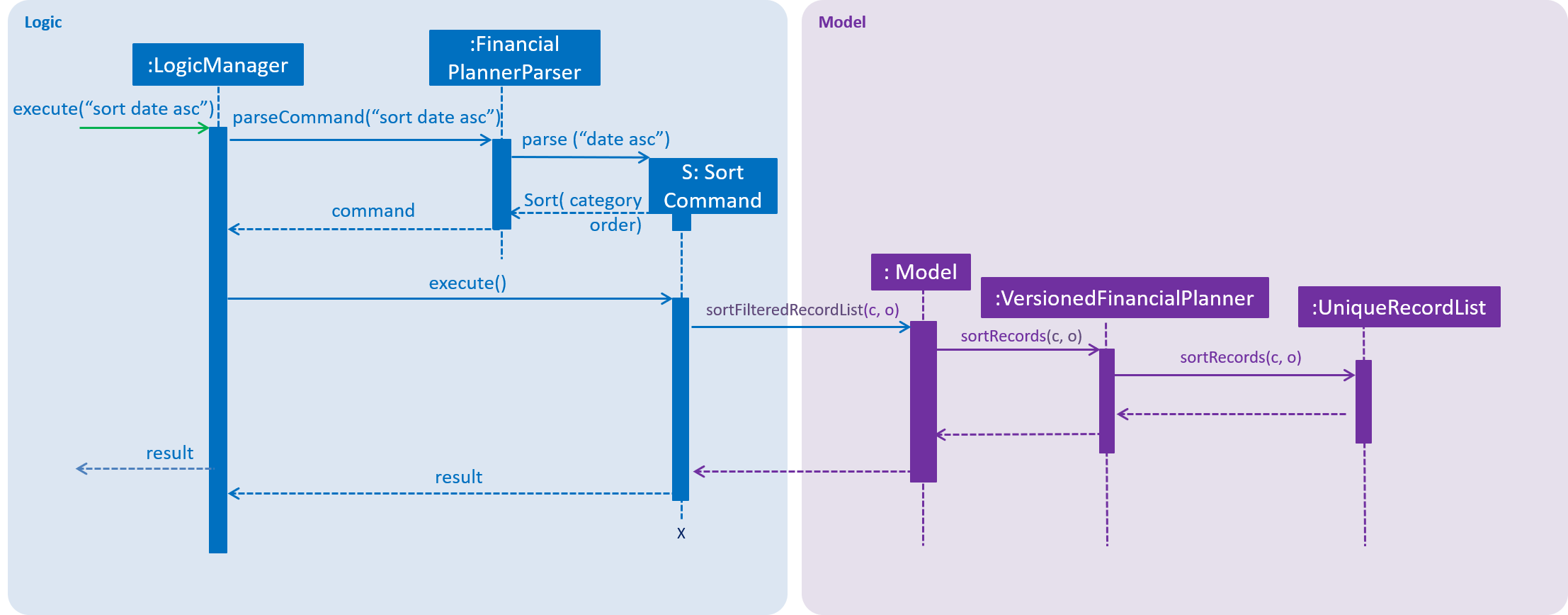
3.4.2. Design Considerations
Aspect: Method of sorting data
-
Alternative 1 (current choice): Sort the observable array list in the underlying data structure in
UniqueRecordList
-
Pros: Easy to implement, sorting will be permanent, user need not sort again with every following command
-
Cons: Not very intuitive, user may not have intended to modify the underlying data arrangement instead only want to sort the data just this once for viewing
-
-
Alternative 2: Sort the FilteredList of records obtained after filtering the underlying array list
-
Pros: Does not allow the user to alter the arrangement of the underlying data, and only obtains a sorted version of the read only data.
-
Cons: Unable to sort a FilteredList as it does not support it, implementations could instead use SortedList but it will not be able to perform the filtering function
-
3.5. View summary feature
This feature allows the user to view a summary table of all their financial activity within a period of time. There are 3 different ways the user can view the summary, one is summary by date which means the summary of each day in the period will be provided, summary by month or summary by category.
The corresponding command required for this feature is summary
and the user will have to supply 2 dates and a compulsory parameter mode
which determines whether
they are viewing summary by date, by month or by category. This feature involves most components of FinancialPlanner with the exception of Storage
. It can also be broadly split into
2 phases, the logic phase which generates the summary and the UI phase which allows users to view the summary in a table.
As shown below, SummaryByDateCommand
, SummaryByCategoryCommand
, SummaryByMonthCommand
is a type of SummaryCommand and thus inherits from SummaryCommand
. The rationale behind this design choice is that
code shared by these classes can be placed in SummaryCommand
for code reuse. This makes it easier for future developers to easily extend this functionality.
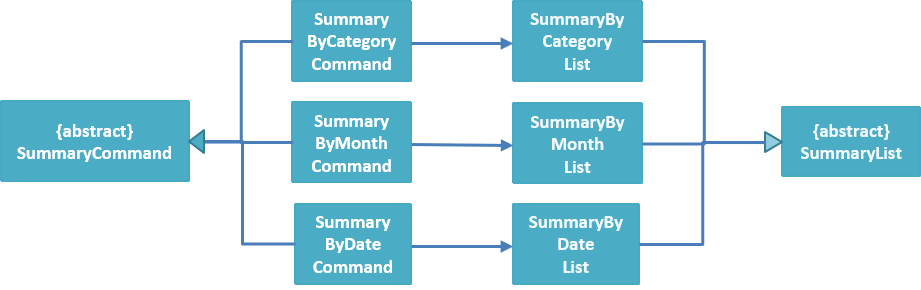
Also, these commands utilise their own version of summary lists which is also inherited from the parent class SummaryList
as shown below. Below details the logic phase.
-
When the user types in the command "summary date d/1-1-2018 12-12-2018", the command is passed from LogicManager to FinancialPlannerParser. In here, the system chooses which parser to use and calls its parse method which is polymorphic, meaning that every parser has the same function but use different implementation.
-
In this class, based on the
mode
parameter given, the system chooses a SummaryCommand to instantiate and pass the reference back to the Logic Manager. The various checks for the validity of the parameters also occur during this stage. -
After the SummaryCommandObject is created,
SummaryByDateCommand#execute
is called. The responsibility of SummaryByDateCommand is a manager that has retrieves information from model and passes it to other components. SummaryByDateCommand gets the filtered list from Model usingModel#getFilteredRecordList
and passes it to the constructor of SummaryByDateList. The createsSummaryList
object which is then passed into aShowSummaryTableEvent
before triggering the event. -
The entire logic process is the same for SummaryByMonthCommand and SummaryByCategoryCommand but uses different lists.
The sequence diagram below details the sequence of program executions for the logic phase.
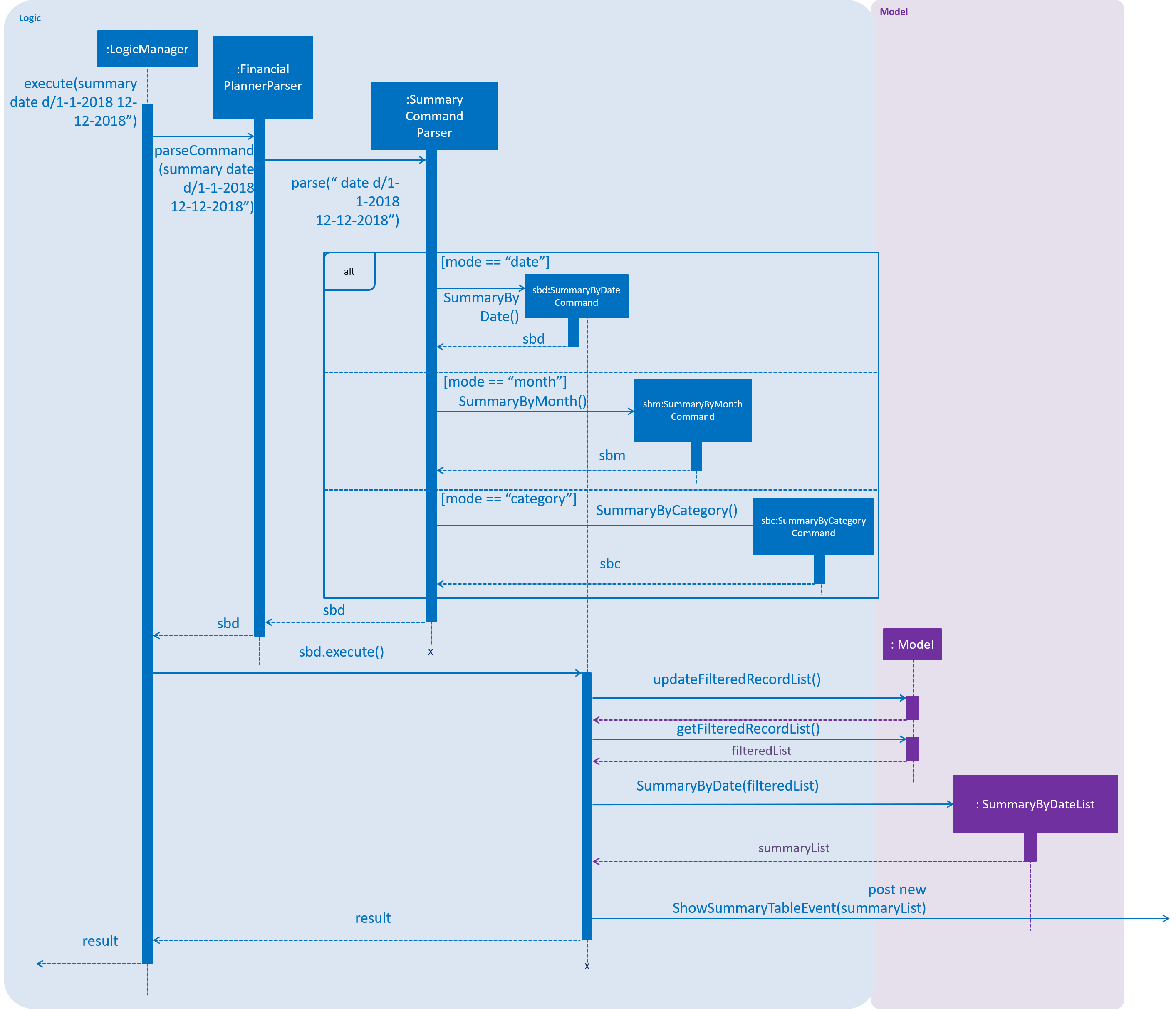
The next phase of the program execution is performed in the UI components.
-
MainWindow
will listen for theShowSummaryTableEvent
and render all Main UI panels invisible before renderingStatsDisplayPanel
visible. -
It then calls the handler function in
StatsDisplayPanel
which will create tabs and call the constructor ofSummaryDisplay
. -
Within the constructor of
SummaryDisplay
, the table is created and the SummaryList is converted into a Ui friendly list.
3.5.1. Design Considerations
Aspect: Method of generating the summary
-
Alternative 1 (current choice): Generate the summary list whenever the summary command is called.
-
Pros: Easier to implement and maintain. Sufficient for the intended target audience of FinancialPlanner.
-
Cons: This requires looping through each record in the filtered record list obtained from the
Model
. To aid in the time complexity, the internal implementation of SummaryList was done using hash maps instead which allowed for constant time random access.. However, the initial filtering is close to linear time complexity which could slow down the app if many records are inside. Also, the list had to be created every timesummary
is called which could be slow if the command is called multiple times.
-
-
Alternative 2: Morph the record list into a record hash map of record lists instead
-
Pros: A hash map allows for constant random access to a record list of a particular date assuming the key for the hash map is using dates. Thus, the filtering function does not need to loop through as many records and the time taken would be lower especially when the database in the application is large.
-
Cons: Might be too specific to only 1 type of category like categorising by date. If any other types are required, another map may have to be added. This implementation may make the system rigid and hard to modify in the future.
-
-
Alternative 3: Cache the summary list in financial planner
-
Pros: By caching the summary list in the financial planner and assigning a boolean variable along with information on the filter predicate to it to determine if it is modified, we can reduce the number of times summary list is recreated every time the
summary
command is called. When thesummary
command is called, it checks the boolean variable to see if summary list needs to be modified. If it doesn’t need to be regenerated, the system will simply read directly fromModelManager
. -
Cons: This implementation involves tracking of the state of the summary list. If it is not done systematically, it may have some hidden bugs which can be hard to test. Also, if the sequence of commands is as follows, summary, add, summary, the time required is still long.
-
3.5.2. Aspect: Method of switching UI panels
-
Alternative 1(current choice): Disable all UI panels within the Main UI placeholder before enabling the desired one
-
Pros: Easy to implement and apply it to other newly created panels. To make use of the current implementation, the panel can simply be the children of the main Ui placeholder and its event handler can be placed in main window.
-
Cons: Might be inefficient when there are many panels or many switching as the same process must be repeated for all panels. However, this is unrealistic and it is unlikely that there are a lot of UI panels for it to make a significant impact.
-
-
Alternative 2: Track which UI panel is visible and only hide that panel
-
Pros: Might have some benefits if the amount of resources available is low
-
Cons: Slightly harder to implement but unlikely to have visible benefits.
-
3.6. View category breakdown feature
3.6.1. Current Implementation
This feature allows the user to view a pie chart breakdown o f all expenses and all income within a date range which the user can specify.
The corresponding command required for this feature is stats
.For this feature, users have to enter 2 dates, one starting date and one ending date.
This feature is facilitated by a few key components of FinancialPlanner, Logic
, Model
, UI
and function executions can be split into 2 phases, the Logic phase
and the UI phase
.
The detailed execution sequence of functions used for Logic phase
are as shown below.
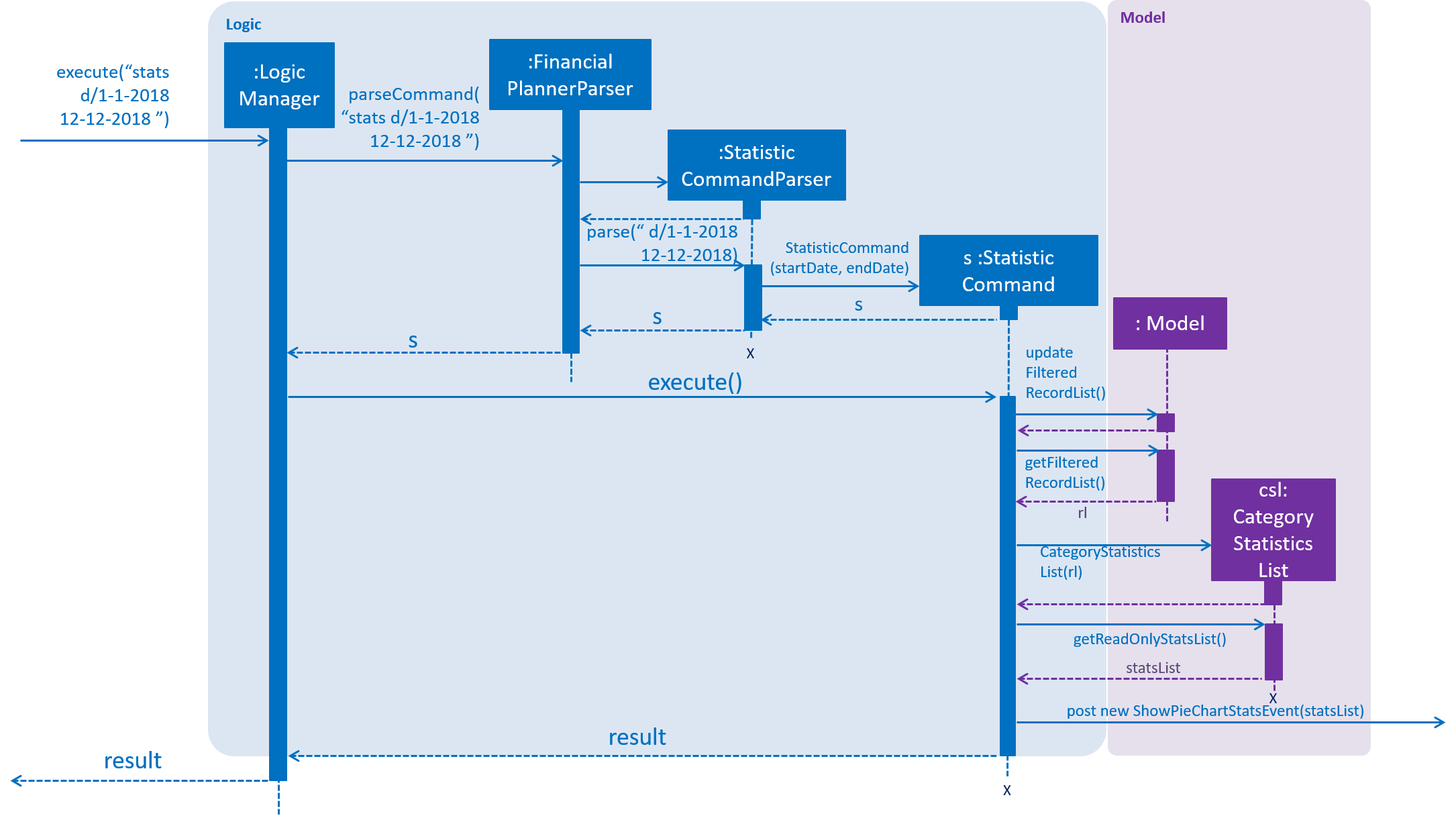
Consider the situation where the user enters "stats d/1-1-2018 12-12-2018":
-
When user enters the command, the
LogicManager
recognises the command and calls theFinancialPlannerParser
to process the new command as shown above. -
The FinancialPlannerParser will then search for the
stats
keyword required and once it is found, the rest of the command minus the keyword is passed in as a parameter to StatisticCommandParser. -
The StatisticCommandParser will then parse the arguments and create a new StatisticCommand object before returning its reference. The activity diagram below details the mechanism within the
StatisticCommandParser#parse
method.
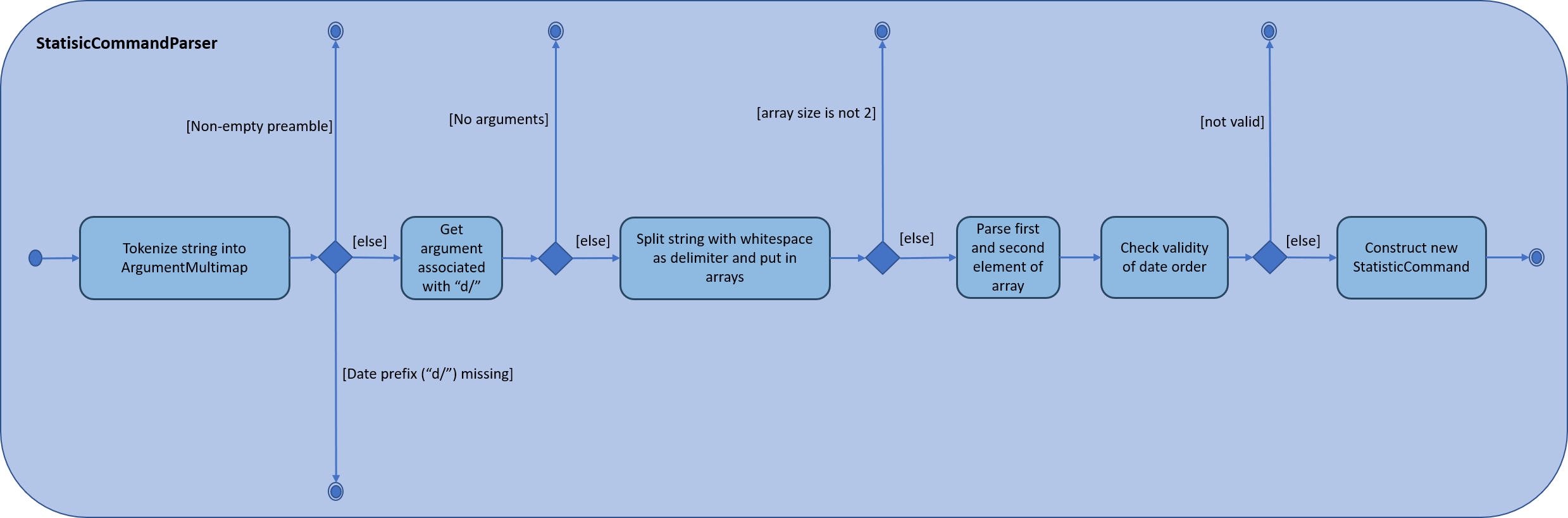
-
Once
StatisticCommand#execute
is called, it will then search through the in-memory data of FinancialPlanner and return a list containing all records within the date range and including both the start dates and end dates. This functionality is facilitated by theModelManager
which is the class that manages all interactions betweenLogic
andModel
component, by`ModelManager#updateFilteredRecords`. The command then retrieves the filteredList from ModelManager and passes it into the constructor ofCategoryStatisticsList
. -
In this constructor, it will loop through all the records in the list and add them into an internal map. The internal data structure in
CategoryStatisticsList
is a hash map, to aid in the adding process, however it only outputs lists and not the map. This functionality is facilitated byCategoryStatisticsList#addToCategoryStatistics
which checks whether the record is in the map. -
If the record is not present, it creates a new
CategoryStatistic
object and adds that to the map. If the record is present, the record is then added to the existingCategoryStatistic
object. -
The flow of control returns to StatisticCommand and StatisticCommand calls the read function of CategoryStatisticsList to obtain a read-only list and passes it into an event constructor before posting the event
ShowPieChartStatsEvent
.
After the event is posted, the execution proceeds to the UI phase
where there is a listener in MainWindow
listening to this event. This is facilitated by
the event system in FinancialPlanner. The sequence diagram below details the program flow of the functions executed in UI phase
for creating the pie chart for total expense.
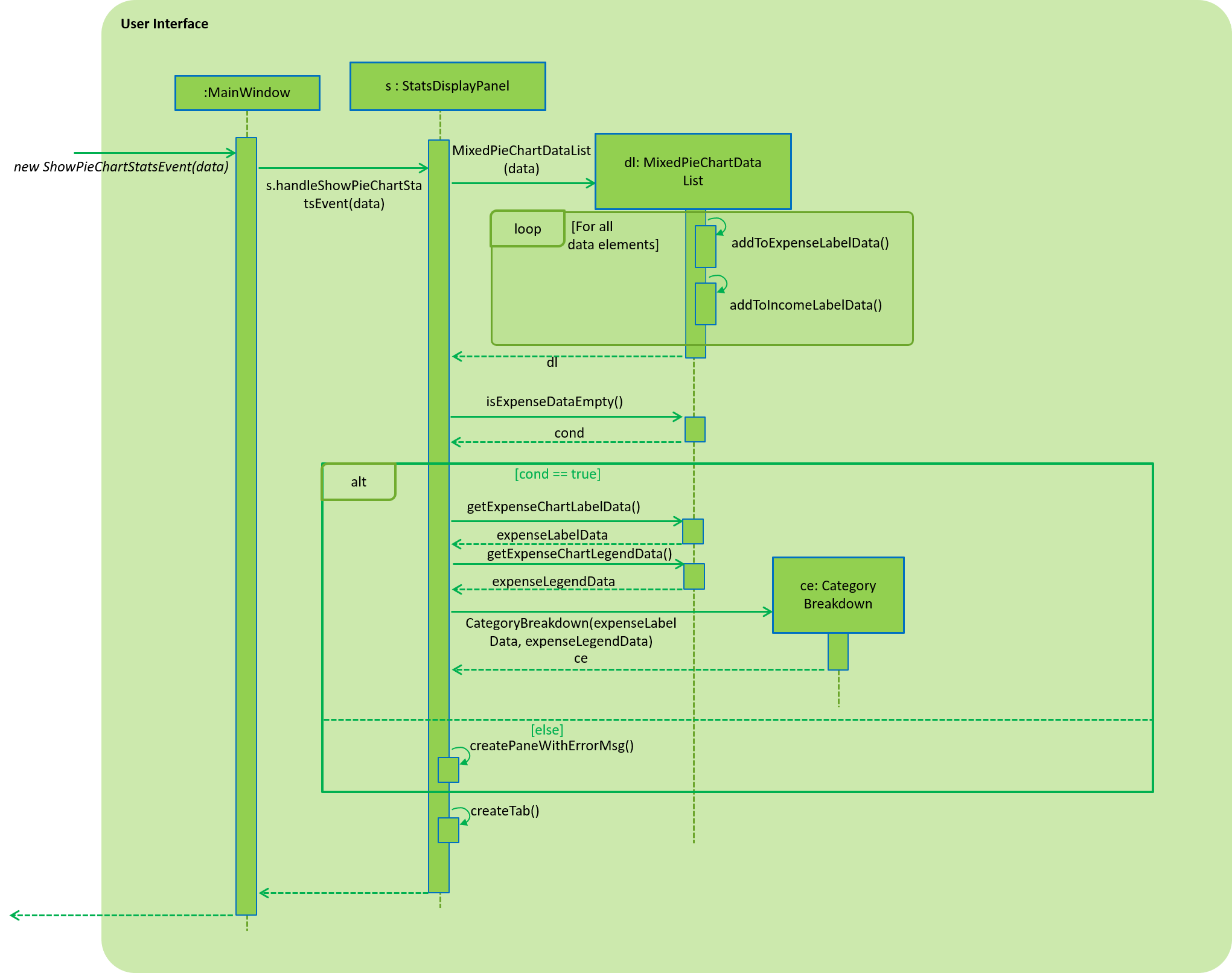
-
When the event is caught by the listener in
MainWindow
,MainWindow
looks through all children of theMainWindow#mainUiPanelPlaceholder
and executes the hide function in them. This will make all children hidden from view in the UI which ensures that the UI is displayed correctly. -
As shown above, the function
StatsDisplayPanel#handleShowPieChartDataEvent
is called which will call the constructor ofMixedPieChartData
. The detailed execution details within this class is as shown in the activity diagram below.

The program flow is then as shown above where the CategoryBreakdown
is created and instantiated with 2 lists, one being expenseLabelData and other being expenseLegendData.
In the current implementation, whenever stats
is called, 2 tabs will be created, one for total income and one for total expense. Thus, the same program sequence after construction for
CategoryBreakdown
is also repeated for total income.
The sequence diagram below details the program flow after the constructor of CategoryBreakdown class is called.
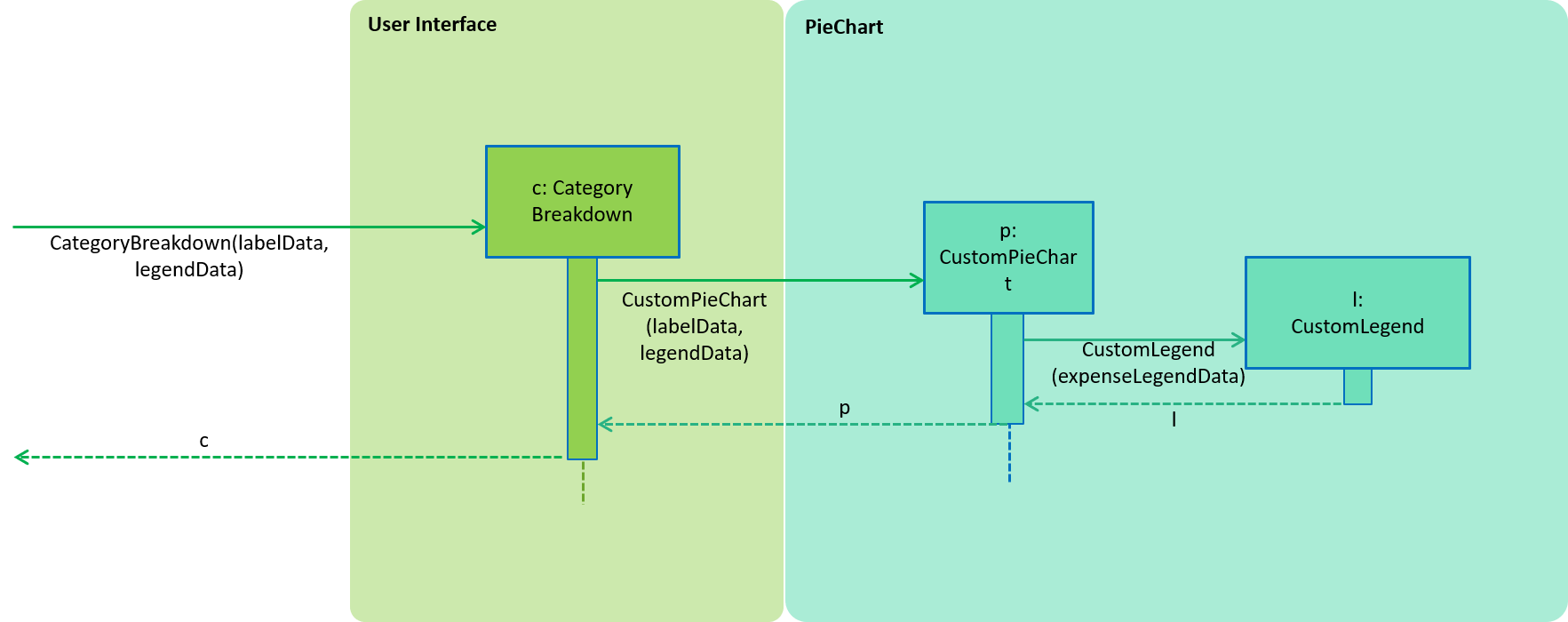
Note that the CustomLegend class is located within the CustomPieChart class which inherits from the JavaFX PieChart class. This legend class can only be accessed within CustomPieChart for security purposes. |
3.6.2. Design Considerations
Aspect: Data structure to support statistic command
-
Alternative 1 (current choice): The pie chart breakdown is regenerated from the filtered list every time this command is called.
-
Pros: Reduce overhead during normal operations like adding, deleting and editing if we do not have to update the statistics in real time.
-
Cons: If the command is called multiple times, this process could be repeated many times which may make the app sluggish when there are many records.
-
-
Alternative 2: Use a data structure to store the statistic information when there is mutation of data
-
Pros: Since the statistics are constantly updated, whenever the command is called, system can read directly and not have to recompute.
-
Cons: Adds overhead to usual operations. Benefits may not be visible if the command is not called frequently.
-
3.7. View current month’s breakdown
This feature does not require user to type any command into the command box or update manually. It provides information about the category breakdown for the current month, presenting income and expense statistics together in one panel. In addition, to improve usability, accelerators were assigned to the menu bar and HOME key is the corresponding accelerator for this feature.
The current implementation of this feature closely follows the observer design pattern. Whenever the system detects changes to the underlying list, like adding, deleting or editing
records, it updates a listener which is attached to the FilteredList
recordsInCurrentMonth present in ModelManager. To check if the current month is the same as the cached month,
the listener will run a check by creating a separate DateIsWithinIntervalPredicate
and comparing it with the current Predicate
of the filtered list.
This listener will then process changes in the list and compute a CategoryStatisticsList
before passing it into the constructor of UpdateWelcomePanelEvent
.
Then the event is triggered, and the corresponding listener on the UI side is called.
The program flow on the UI side is similar to that of viewing category breakdown of a time period with the exception that tabs are not created.
The accelerators and the menu bar is connected to the UI component and will trigger MainWindow#handleHome
whenever the accelerator HOME key or Home is selected using the menu.
3.8. Find records by tag feature
3.8.1. Current Implementation
The findtag mechanism is also facilitated by ModelManager
. FindTagCommand calls ModelManager#updateFilteredRecords
and passes in different predicates depending on the input by the user.
This feature has only one keyword findtag
and a single working mode which takes in any number of input arguments. The
input given by the user is passed to FindTagCommandParser#parse
to split the desired tags the user wants to search by
into an array of strings. The array of strings is passed into TagsContainsKeywordsPredicate
to create the predicate
for updateFilteredRecordList
required in ModelManager
.
In TagsContainsKeywordsPredicate
, to compare for a match, every keyword in the array is compared
against the set of tags of each record and as long as any tag matches any of the keywords,
the predicate will evaluate to true and allows the FilteredList
to filter out the records that do not fulfil the
predicate.
FindTagCommandParser
returns a FindTagCommand
object which calls updateFilteredRecordList
to set the new predicate
and obtain a new filteredRecords
based on the predicate, which will also trigger an event for the UI to read in and display the new records.
The following sequence diagram shows how the limit operation works:
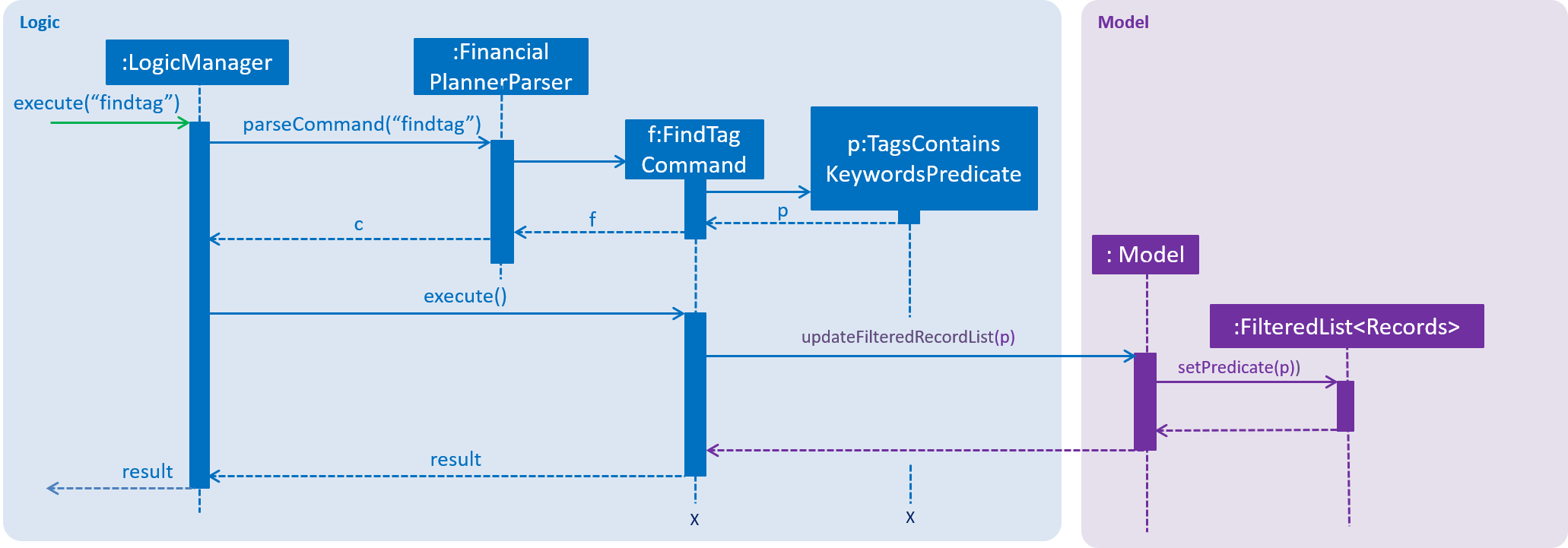
3.9. Limit features
-
Limit features' storage:
-
The limit features are based on the data type
Limit
, which includes twoDate
s and onemoneyFlow
. When entering only one date, the parser will let the date be both dateStart and dateEnd. It is equivalent to entering two same dates. -
DateStart will always be earlier than or equal to dateEnd.
-
The limit storage is based on the
Date
. More than one limit for the same period of time is not allowed.
-
-
Limit features’s check:
-
Whenever the user change the recordList information, including adding a record, deleting a record and editing a record, all the limits will be checked automatically by calling the function
autoLimitCheck()
. -
The
autoCheckLimit()
function will look through all the records, calculate the total money for each limit , generate a string which contains all the exceeded limits' information and print the string out to warn the user. -
To get the output, the function will execute a loop, which will execute the
isExceeded()
,getTotalSpend()
andgenerateLimitOutput()
for every limit and combine all the limits' output into one string.
-
-
Limit features' MoneyFlow:
-
Unlike the moneyFlow used by addCommand, the limit moneyFlow input can only be normal real number, which does not have "-" or "+" in front of the number. For example,
m/500
. -
After user input the normal real number, the parser will add a "-" at the beginning of the real number, which makes it a normal moneyFlow.
-
If user input wrong form of limit moneyFlow, the program will throw an error.
-
-
Limit features' parsers:
-
All the limitCommand Parsers are similar to each other. However, there are some differences between different commands. Detailed information will be provided in specific commands.
-
The input given by the user is passed to
ArgumentTokeniser#tokenise
to split the input separated by prefixes. -
This returns a
ArgumentMultiMap
which contains a map with prefixes as keys and their associated input arguments as the value. -
The string associated with
m/
will be checked. If the form is correct. If the form is wrong, the program will throw an error, otherwise it will be constructed as aMoneyFlow
type. -
The string associated with
d/
is then passed intoxxxLimitCommandParser#splitByWhitespace
for further processing and returns an array. This string will be split into two strings and each of them will be constructed as aDate
type variable. If there is only one date string, this date will be set to both dateStart and dateEnd. -
After parsing the two dates, the parser will check whether the dateStart is earlier than dateEnd.
-
Lastly a new limit will be generated with the dateStart, dateEnd and money and return the xxxLimitCommand with the limit.
-
-
-
Monthly Limit:
-
The addMonthlyLimit command is to add a continuous limit always for the current month. The limit will always check the spend of the month according to the current time. For example, if the limit was set to be 200 at April, the limit will check the total spend for April. When the time comes to May, the limit will no longer check April, instead, the limit will check the total spend of May until the last second of May.
-
The user will input only the money they want to set.
-
The parser will make the limit with a special date
01-01-9999
, which is not likely to be used. -
Once the monthly limit is going to be checked, the function
generateThisMonthLimit()
will be called, which will generate a temporary limit according to the current date. Then use this temporary limit to do the limit check and generate output.
-
3.9.1. addLimit feature
Current Implementation
This command is to add a new limit according to the dates input.
The command will read in a limit and store the limit by calling the addLimit()
function.
The user enters two dates (or one date) after the one "d/" index followed by money with m/ index.
-
If there is already a limit with the exactly same dates, the program will throw an error and the limit can not be added.
The following sequence diagram shows how the limit operation works:
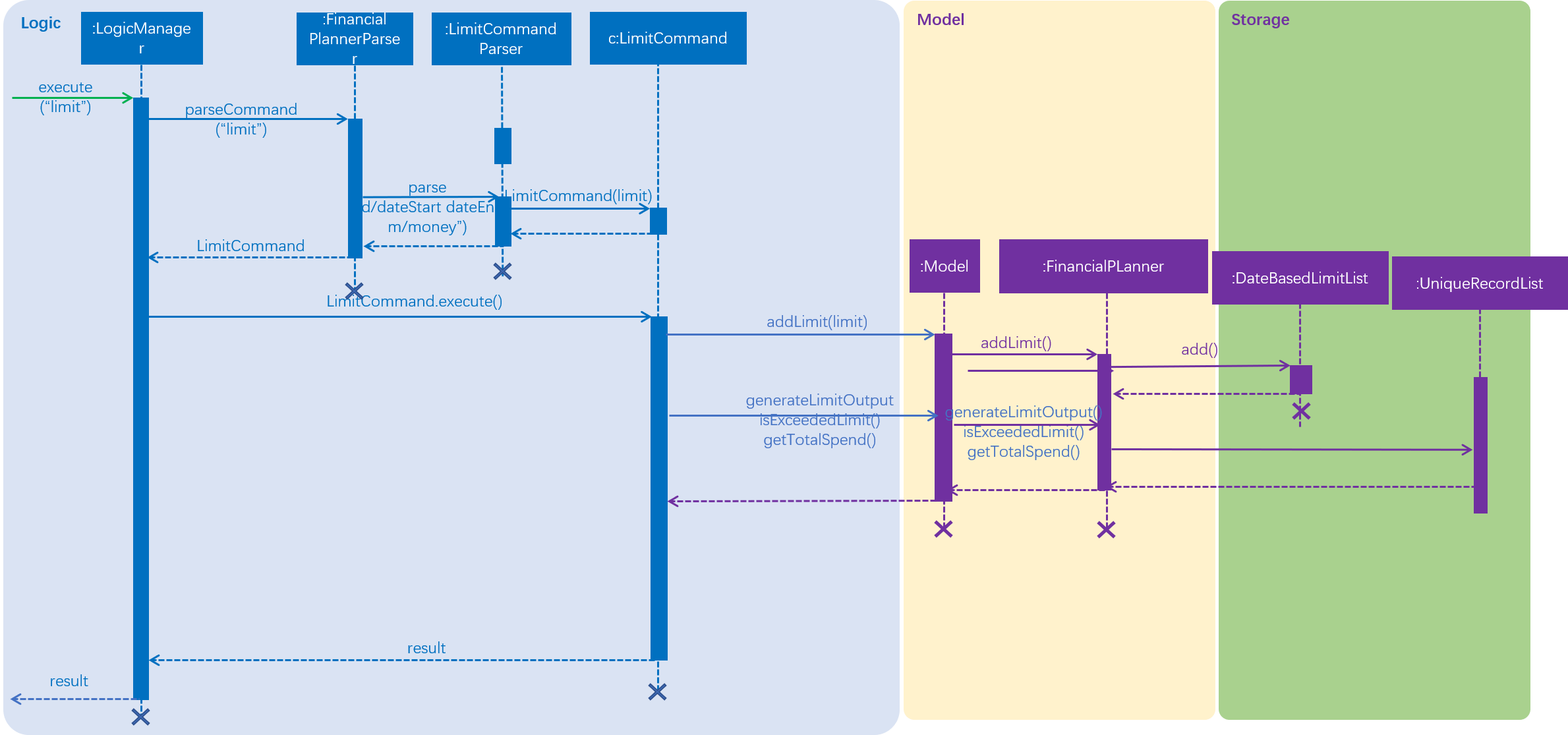
3.9.2. deleteLimit feature
Current Implementation
This command is to delete an existing limit.
The command will read in a limit with the input dates and delete the limit with the same dates by calling the deleteLimit
function.
The user enters two dates (or one date) after the one "d/" index.
-
The deleteLimitCommandParser will use the dates and a dummy valid moneyFlow to make it a complete limit.
-
If there is no limit with the same dates, the program will throw an error.
The following sequence diagram shows how the deleteLimit operation works:
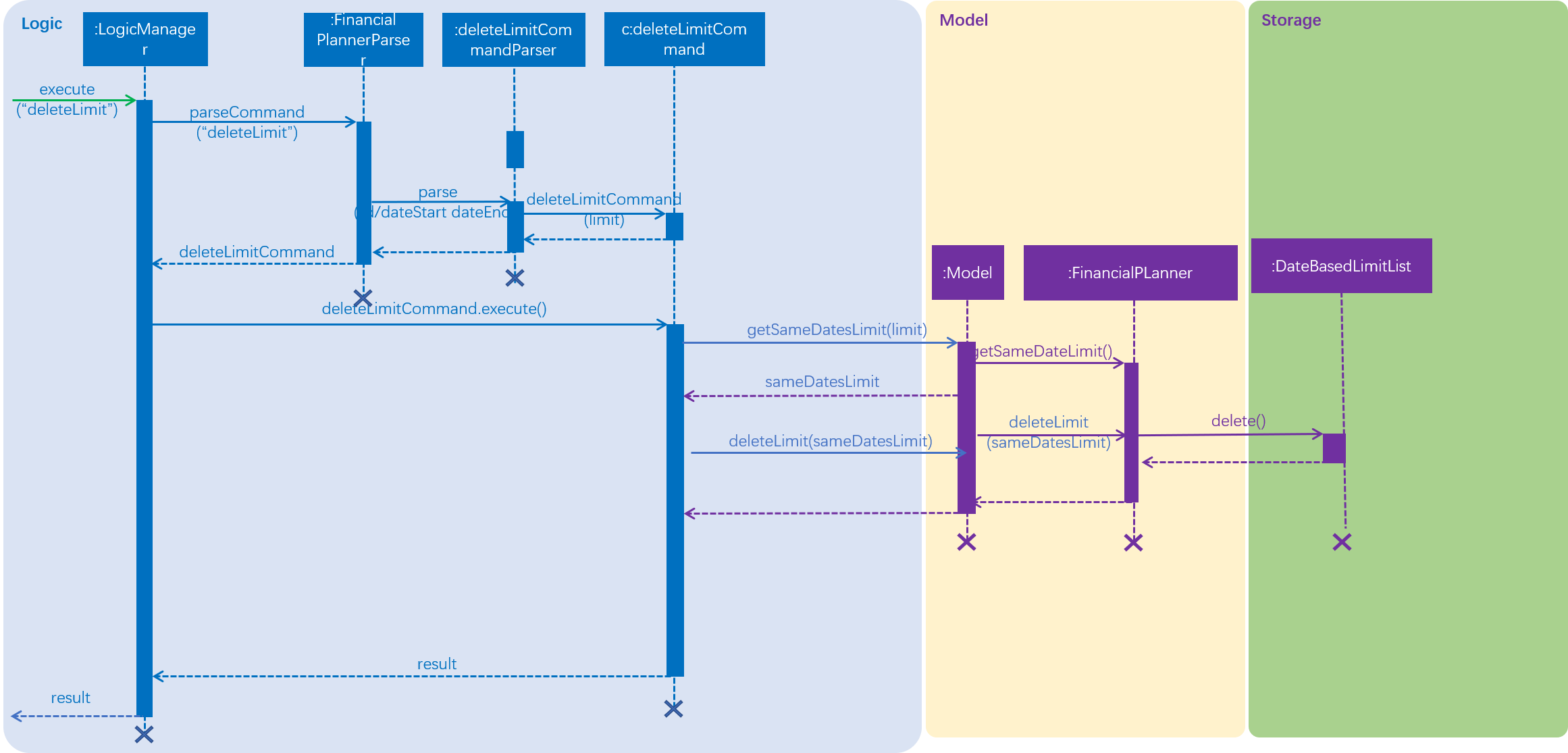
3.9.3. editLimit feature
Current Implementation
The editLimit command is to edit an existing limit.
The command will read in a limit and replace the limit with the same dates by calliing the updateLimit()
function.
The user enter two dates after the one "d/" index followed by money with m/ index.
-
If there is no limit with the same dates, the program will throw an error.
The following sequence diagram shows how the limit operation works:
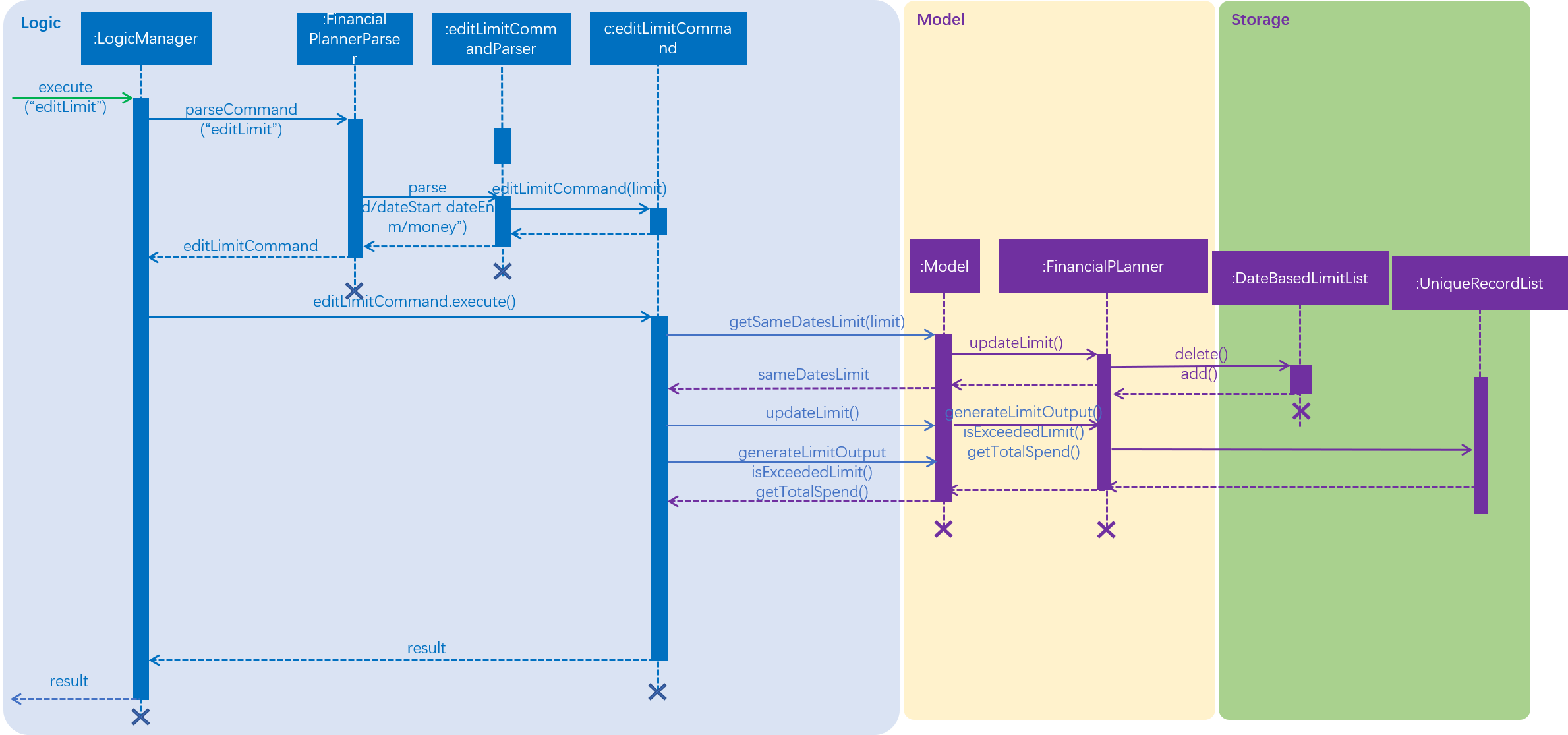
3.9.4. addMonthlyLimit feature
Current Implementation
The addMonthlyLimit command is to add a monthly limit.
The command will read in the monthly limit and store it by calling the addLimit()
function.
The user only enter money after the one "m/" index.
-
The parser will use the special date and the input money to make a complete limit.
-
If there is already a monthly limit, the program will throw an error.
The following sequence diagram shows how the limit operation works:
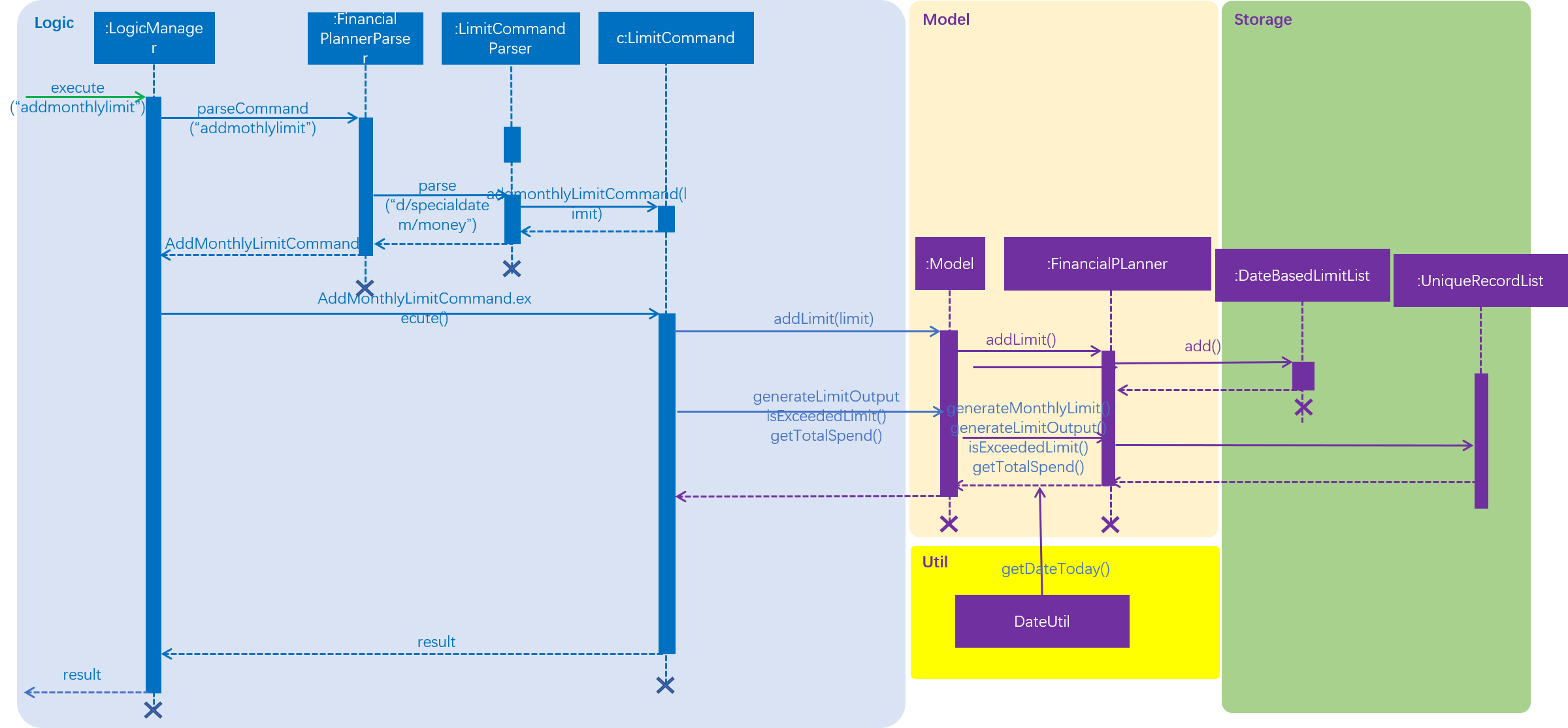
3.9.5. editMonthlyLimit feature
Current Implementation
The editMonthlyLimit command is to edit the monthly limit.
The command will read in the monthly limit and update the monthly limit by calling the updateLimit()
function.
The user only enter money after the one "m/" index.
-
The parser will use the special date and the input money to make a complete limit.
-
If there is no monthly limit, the program will throw an error.
The following sequence diagram shows how the limit operation works:
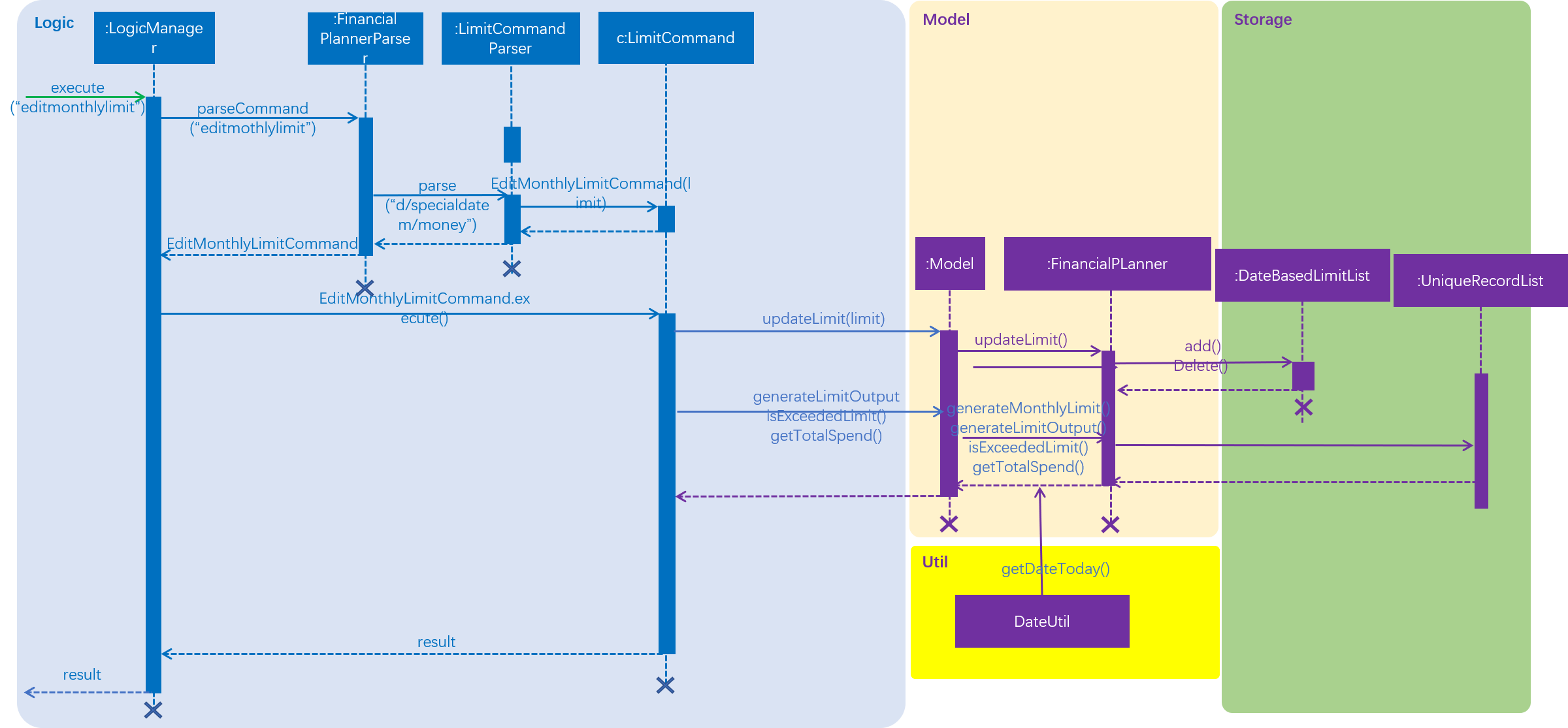
3.9.6. deleteMonthlyLimit feature
Current Implementation
The deleteMonthlyLimit command is to delete the monthly limit.
The command will read in the monthly limit and update the monthly limit by calling the deleteLimit()
function.
The user will only enter the command word.
-
The parser will use the special date and a dummy moneyFlow to make a complete limit.
-
If there is no monthly limit, the program will throw an error.
The following sequence diagram shows how the limit operation works:
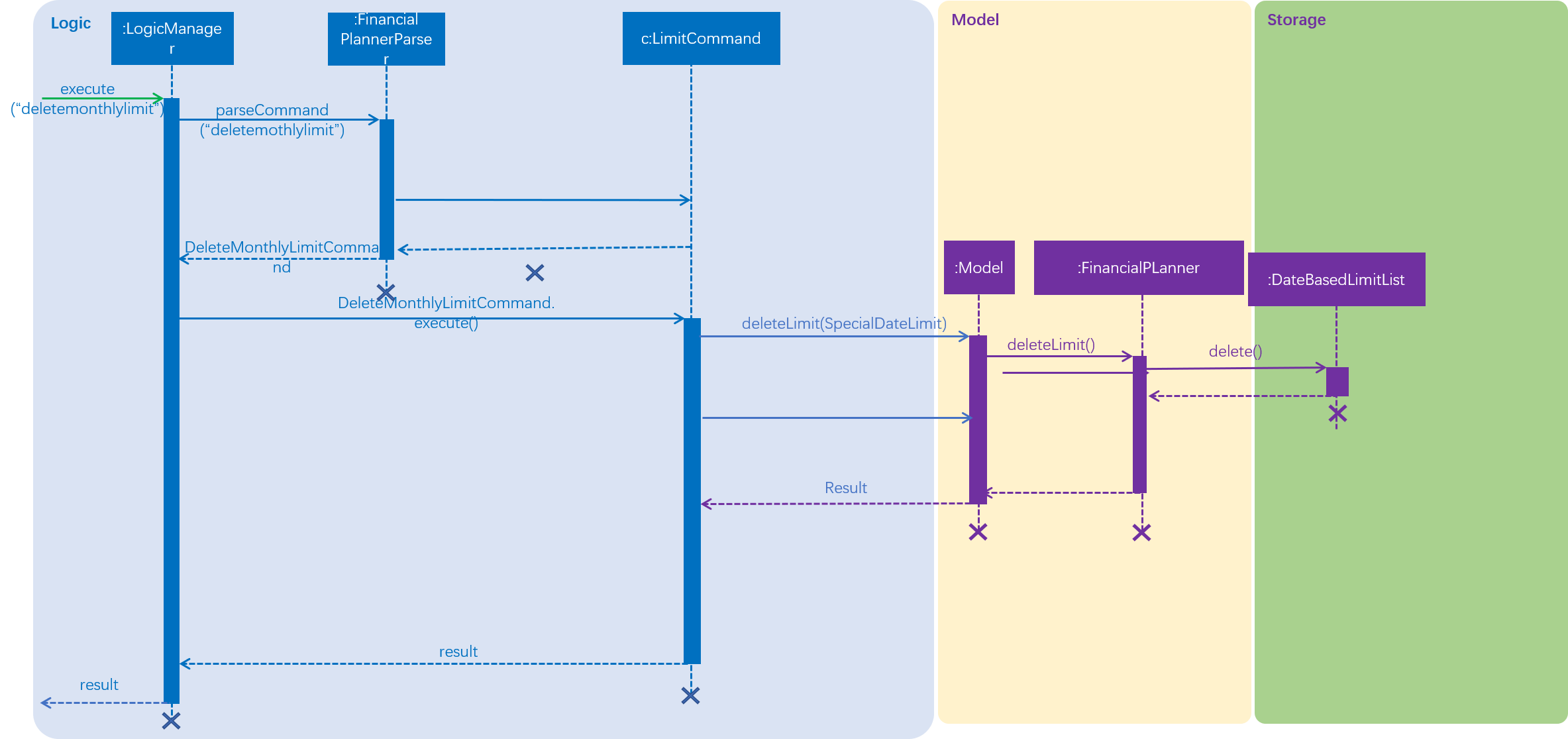
3.9.7. checkLimit feature
Current Implementation
This feature is to help the user to check all the limits stored inside the limitList. The function will call manualLimitCheck(), which will generate a string that contains all limits' information.
-
When there is no limits inside the limitList, the program will throw an error.
The following sequence diagram shows how the limit operation works:
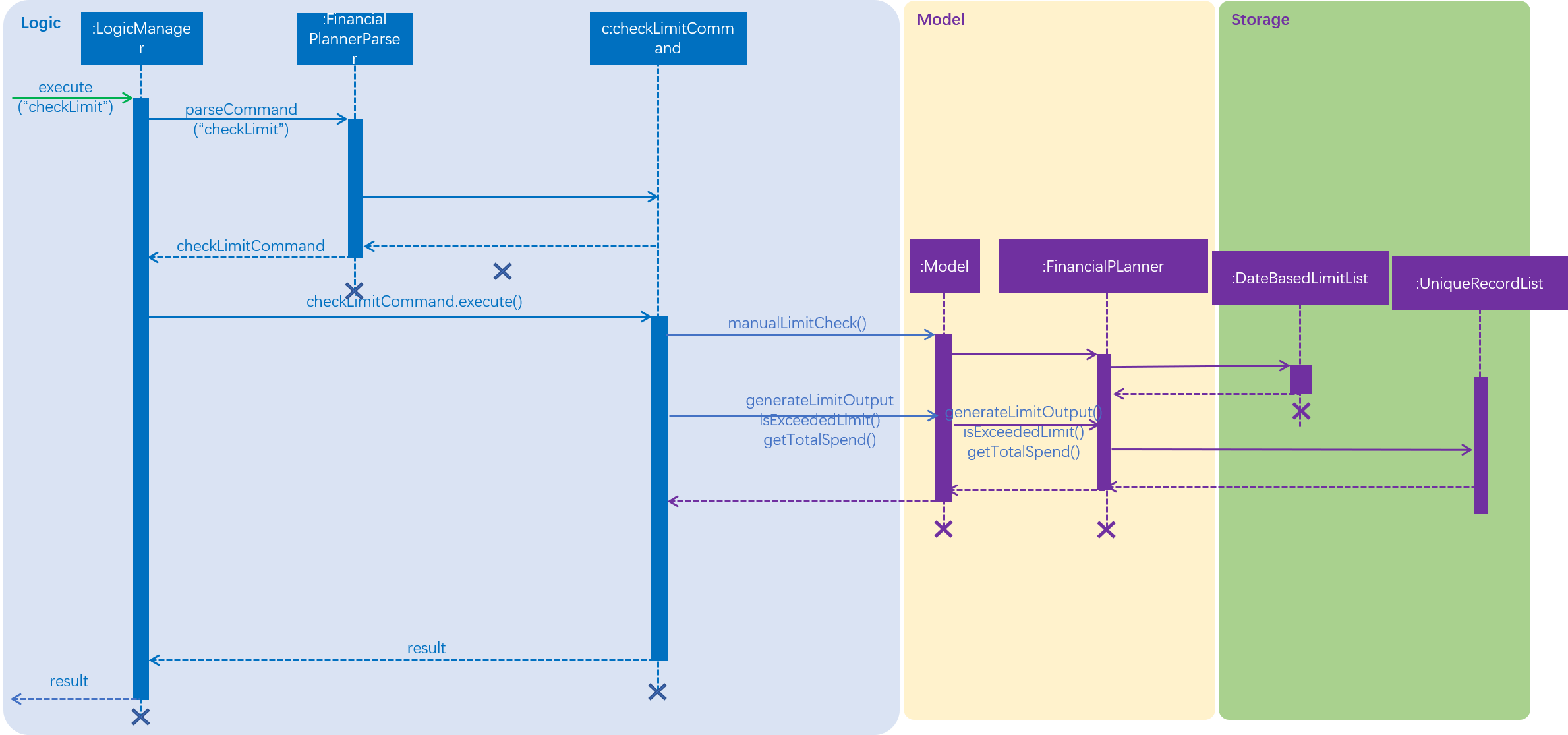
3.10. Delete all the record whose date is required
3.10.1. Current implementation
The delete by date entry mechanism is facilitated by ModelManager
.
It represents an in-memory model of Savee and is the component which manages the interactions between the commands and the VersionedFinancialPlanner
. DeleteByDateEntryCommand calls ModelManager#getFilteredRecordList
to retrieve the list of all current records in the Savee. Then, it will loop through the list of records and call ModelManger#deleteRecord(Record record)
to delete the record whose date is required. If there exists target records, ModelManager#commitFinancialPlanner
will be called to update the current version of Savee and the message, which states records have been deleted. Then, ModelManager#autoLimitCheck
will be called to check the current change in limit as we delete some records exceeds the limit or not. This feature has only one keyword deletedate
and implements only 1 argument mode.
The three argument modes are as listed below:
-
Single Argument mode — Requires only one date. It deletes all records whose date is required. The date must follow the format: dd-mm-yyyy, error will be thrown if the format is not correct or the date entered is not real.
The following sequence diagram shows how the list operation works:
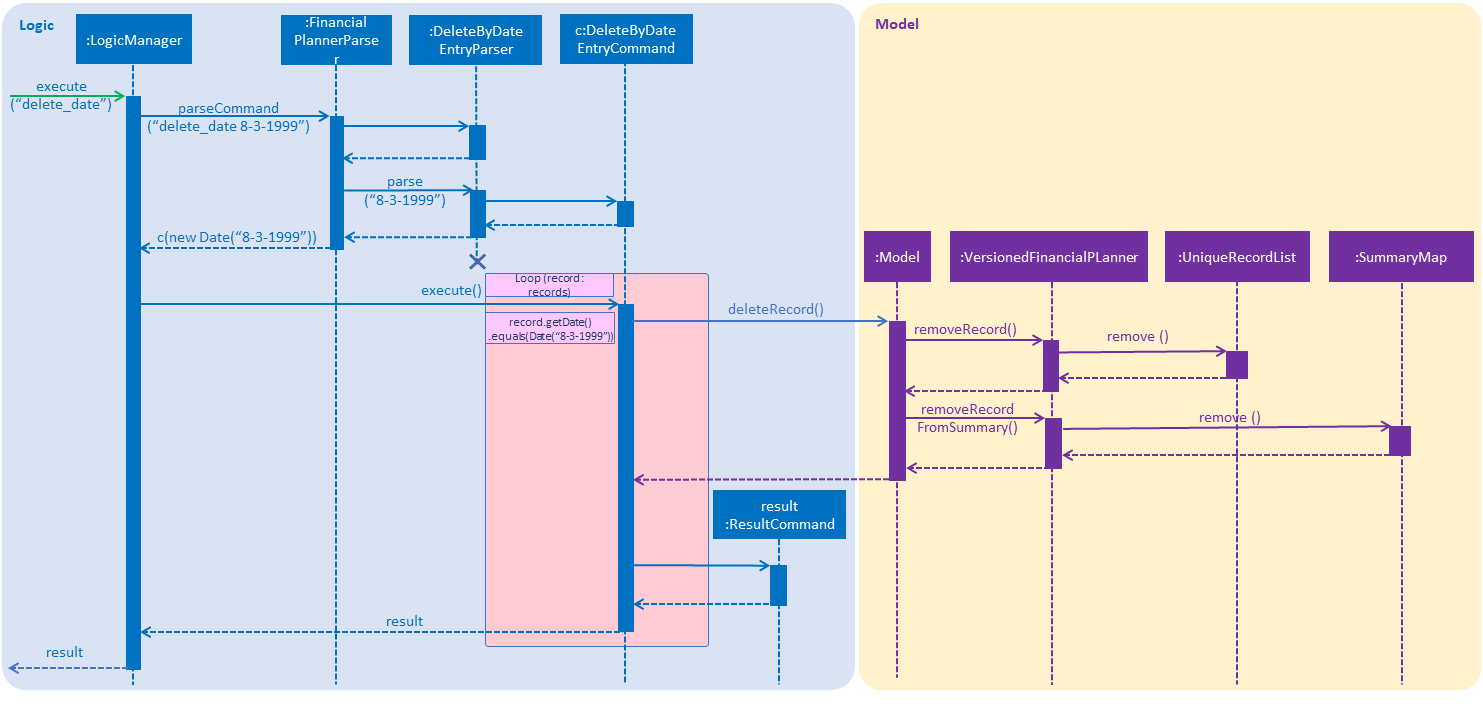
3.11. Export the records from Savee to the Excel file.
3.11.1. Current implementation
The export into excel file mechanism is facilitated by ModelManager
with the help of ExcelUtil
, the utility created to handle all methods relating to Excel. It represents an in-memory model of the Savee and is the component which manages the interactions between the commands, ExcelUtil
and the VersionedFinancialPlanner
. ExportExcelCommand calls ModelManager#updateFilteredRecords
and passes in different predicates depending on the argument mode. The List<Record> is retrieved by calling ModelManager#getFilteredRecordList
. Meanwhile, it also called ModelManager#getFinancialPlanner
to get the ReadOnlyFinancialPlanner
. The SummaryByDateList is constructed after the ReadOnlyFinancialPlanner together with the predicate are passed into the construction of SummaryByDateList. The List<SummaryEntry> is easily retrieved from SummaryByDateList by calling SummaryByDateList#getSummaryList
. ExcelUtil#setNameExcelFile
is called to make the Excel name based on the condition of startDate and endDate. After that, ExcelUtil#setPathFile
is called to set the Path file, which is the location of the Excel file stored in future. The Path file is constructed based on the name of the Excel file we retrieve above and the directory Path, it can be either optionally entered by the user or the default User’s Working Directory. With the sufficient information, List<Record> records
, List<SummaryByDateEntry> summaryList
, file path
, ExportExcelCommand#exportDataIntoExcelSheetWithGivenRecords
is called to start the processing of producing Excel file.
There are 6 modes for this feature [refer to Export the record data from Savee to the Excel file part in User Guide]. The mechanism that facilitates these modes can be found in the ExportExcelCommandParser#parse
. Below is a overview of the mechanism:
-
Method
ExportExcelCommandParser#createExportExcelCommand
takes the input argument and further analyse it. -
The input given by the user is passed to
ArgumentTokeniser#tokenise
to split the input separated by prefixes. -
This returns a
ArgumentMultiMap
which contains a map with prefixes as keys and their associated input arguments as the value. -
The string associated with
d/
-
It is then passed into
ExportExcelCommandParser#splitByWhitespace
for further processing and returns an array. This string will be split into sub-strings and each of them will be construct as a date type variable. The the size of the array exceed 2, error wil be thrown to inform invalid command format. If the size of the string equals 1, it is constructed as a date type variable after being passed toParseUtil#parseDate
, it must follow the format dd-mm-yyyy. Error will be thrown if the format is not correct or the date entered is not real. If the size of the string equals 2, each sub-string is constructed as a date type variable after being passed toParseUtil#parseDate
, and an additional check is conducted to check if the first date entered, known as Start date is smaller than or equal to the second date entered, known as End Date.
-
-
The String associated with
dir/
-
It is then passed into
ParseUtil#parseDirectoryString
to check if the Directory path given is existing. If the Directory path is unreal, an error is thrown to inform the user.
-
-
Please take note that:
-
If the prefix
d/
is not entered in the input, meaning that all the records will be included in the Excel sheet. -
If the prefix
dir/
is not entered in the input, meaning that the Directory Path is default as the User’s Working Directory.
-
The ExportExcelCommand
has four constructors which makes use of overloading to reduce code complexity.
-
One constructor has no arguments and assigns default predicate for the
FilteredList
inModelManager
,PREDICATE_SHOW_ALL_RECORDS
which will show all items in the list and the Directory path is User’s Working Directory. -
The second constructor takes in 2
Date
arguments and assigns the predicateDateIsWithinDateIntervalPredicate
which will only show items within the date interval and the Directory path is User’s Working Directory. -
The third constructor takes in 1
Directory Path
argument and assigns the predicate asPREDICATE_SHOW_ALL_RECORDS
, which will show all items in the list and the Directory path is the entered directory path. -
The fourth constructor takes in 1
Directory Path
and 2Date
arguments and assigns the predicate asDateIsWithinDateIntervalPredicate
which will only show items within the date interval and the Directory path is the entered Directory Path.
The following sequence diagram shows how the list operation works:
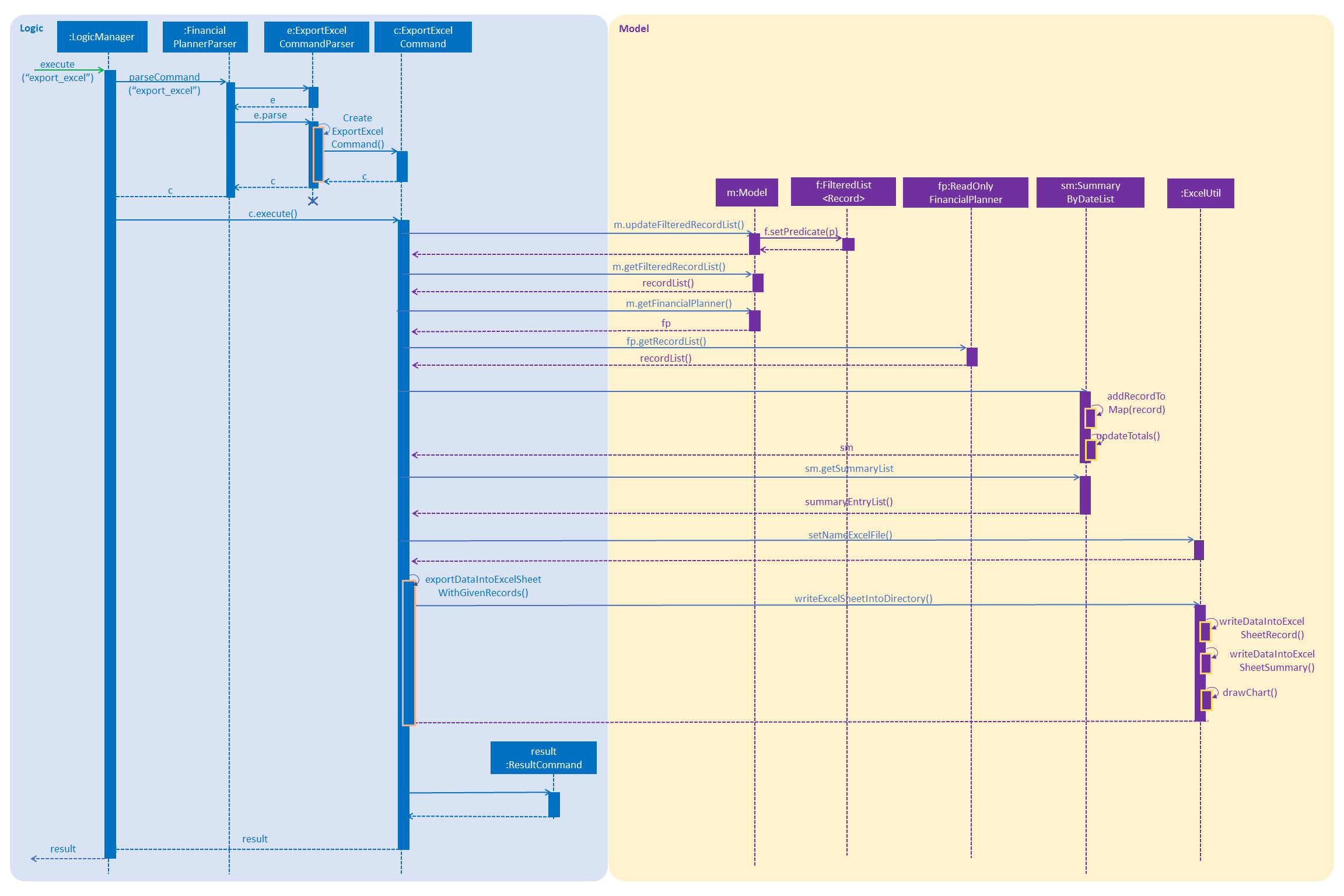
If the Excel file with the same name and stored in same Directory exists, it will be overwritten. However, it must be closed before we enter the command.
Please note that undo
and redo
command can only affect Savee but the not the Excel file created, meaning that when you enter undo
command after you enter the export
command, Savee will inform the user that No more command to undo, the records remain the same and the Excel file created will not be deleted.
3.12. Import the record data from the Excel file to Savee
3.12.1. Current implementation
The import from Excel file mechanism is facilitated by ExcelUtil
, the utility created to handle all method relating to Excel. ImportExcelCommand calls ExcelUtil#readExcelSheet
to read the Excel file and retrieve data of records from them to create a list of record List<Record>. In ExcelUtil#readExcelSheet
, by using method XSSFWorkbook#setMissingCellPolicy
, every missing cell will be considered as Null cell, quite useful when you export or archive the record data if the record does not have tag. If blank row is found, the ExcelUtil#readExcelSheet
will ignore and read the next line, until the last line is read. This concept also applies to read multiple sheets until all sheets have been read. However, please take note that in order to prevent errors, there are certain constraints of Excel file list in User Guide with Import the record data from the Excel file to the Savee part. After that, ModelManager#addListUniqueRecord
is called to add the records in the List<Record>. If the record has already existed in the Savee, it will be ignored and not added into the Savee. Eventually, when all the records are added in the Savee, ModelManager#commitFinancialPlanner
will be called to update the current version of Savee and the message, which states records have been added.
This feature has 2 different modes [refer to in Import the record data from the Excel file to the Savee part in User Guide]. The mechanism that facilitates these modes can be found in the ImportExcelCommandParser#parse
. Below is a overview of the mechanism:
-
Method
ImportExcelCommandParser#createArchiveCommand
takes the input argument and further analyse it. -
The input given by the user is passed to
ArgumentTokeniser#tokenise
to split the input separated by prefixes. -
This returns a
ArgumentMultiMap
which contains a map with prefixes as keys and their associated input arguments as the value. -
The String associated with
dir/
-
It is then passed into
ParseUtil#parseDirectoryString
to check if the Directory path given is existing. If the Directory is unreal, an error is thrown to inform the user.
-
-
The String associated with
n/
-
It is then passed into
ExcelUtil#getPathFile
together with the String associated withdir/
to create the File Path. The File Path is then passed intoParseUtil#parseFilePathString
to check if the File path given is existing. If the File Path is unreal, an error is thrown to inform the user.
-
-
Please take note that:
-
The String associated with
dir/
must be entered. -
The String associated with
n/
is optionally entered. -
Please note that User have to add the post-fix
.xlsx
at the end to indicate this is a Excel file.
-
-
If the string associated with
n/
, methodExcelUtil#setPathFile
is called to combine the name of the Excel file to the string associated withdir/
.
The ImportExcelCommand
has only one constructor.
-
The constructor takes in file path argument and read the Excel file using that file path. After that, all the record data in the Excel file is retrieved and transform into Record object. The list of records are then added into the Model by using
ModelManager#addListUniqueRecord
The following sequence diagram shows how the list operation works:
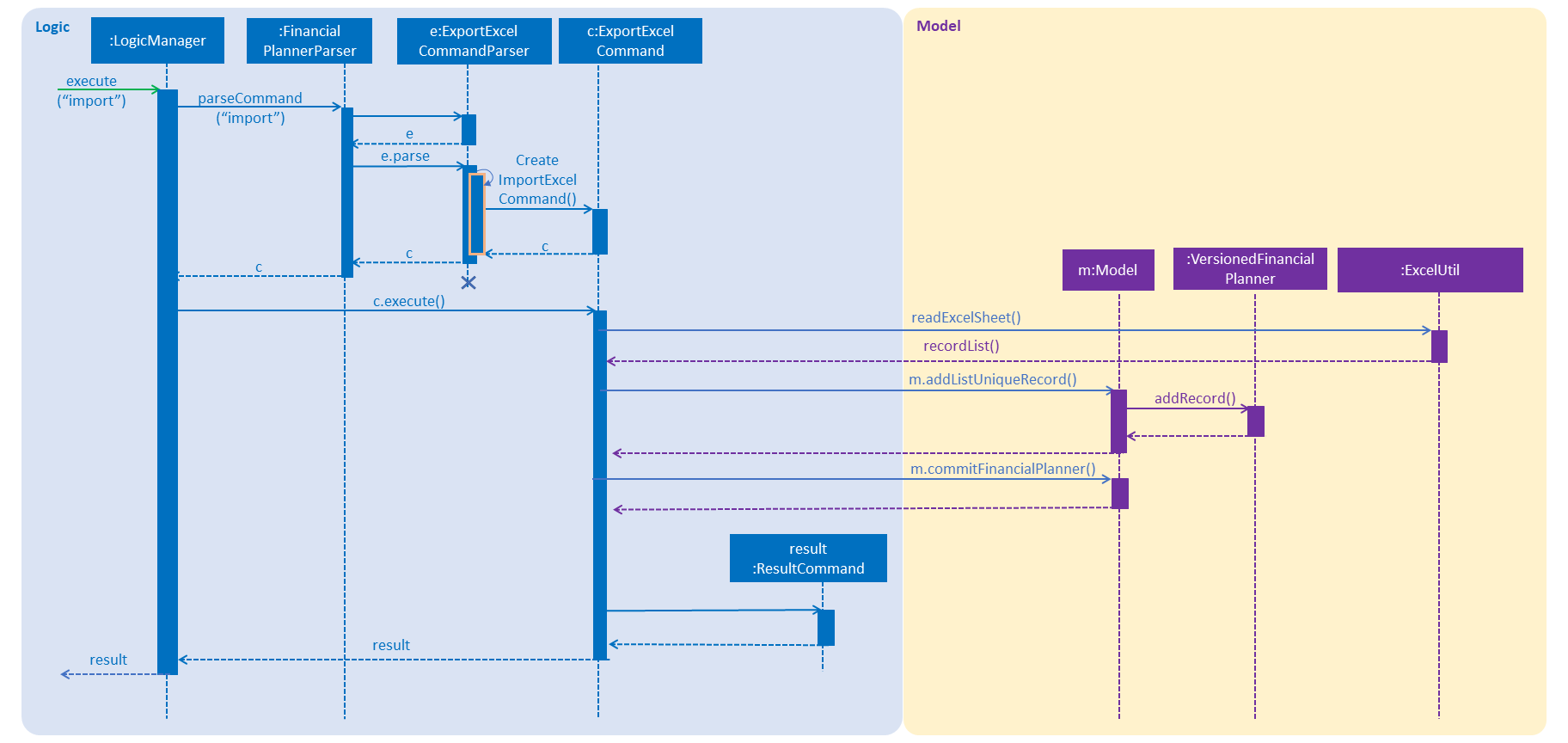
The file user want to import must be closed before entering the command.
Please note that the undo
and redo
command can only affect the Savee but the not the Excel file created, meaning that when you enter undo
command after you enter the import
command, the records imported will be deleted.
3.13. Archive the record data from Savee to the Excel file
3.13.1. Current implementation
The archive into excel file mechanism is facilitated by ModelManager
with the help of ExcelUtil
, the utility created to handle all methods relating to Excel. It represents an in-memory model of Savee and is the component which manages the interactions between the commands, ExcelUtil
and the VersionedFinancialPlanner
. ArchiveCommand calls ModelManager#updateFilteredRecords
and passes in different predicates depending on the argument mode. The List<Record> is retrieved by calling ModelManager#getFilteredRecordList
. Meanwhile, it also called ModelManager#getFinancialPlanner
to get the ReadOnlyFinancialPlanner
. The SummaryByDateList is constructed after the ReadOnlyFinancialPlanner together with the predicate are passed into the construction of SummaryByDateList. The List<SummaryEntry> is easily retrieved from SummaryByDateList by calling SummaryByDateList#getSummaryList
. ExcelUtil#setNameExcelFile
is called to make the Excel name based on the condition of startDate and endDate. After that, ExcelUtil#setPathFile
is called to set the Path file, which is the location of the Excel file stored in future. The Path file is constructed based on the name of the Excel file we retrieve above and the directory Path, it can be either optionally entered by the user or the default User’s Working Directory. With the sufficient information, List<Record> records
, List<SummaryByDateEntry> summaryList
, file path
, ArchiveCommand#archiveDataIntoExcelSheetWithGivenRecords
is called to start the processing of producing Excel file. After records are archived to Excel file, ModelManager#deleteListRecord
is called to delete the archived records.If there exists target records, ModelManager#commitFinancialPlanner
will be called to update the current version of Savee and the message, which states records have been deleted.
This feature has 6 different modes [refer to Archive the record data from Savee to the Excel file in User Guide]The mechanism that facilitates these modes can be found in the ArchiveCommandParser#parse
. Below is a overview of the mechanism:
-
Method
ArchiveCommandParser#createArchiveCommand
takes the input argument and further analyse it. -
The input given by the user is passed to
ArgumentTokeniser#tokenise
to split the input separated by prefixes. -
This returns a
ArgumentMultiMap
which contains a map with prefixes as keys and their associated input arguments as the value. -
The string associated with
d/
-
It is then passed into
ArchiveCommandParser#splitByWhitespace
for further processing and returns an array. This string will be split into sub-strings and each of them will be construct as a date type variable. The the size of the array exceed 2, error wil be thrown to inform invalid command format. If the size of the string equals 1, it is constructed as a date type variable after being passed toParseUtil#parseDate
, it must follow the format dd-mm-yyyy. Error will be thrown if the format is not correct or the date entered is not real. If the size of the string equals 2, each sub-string is constructed as a date type variable after being passed toParseUtil#parseDate
, and an additional check is conducted to check if the first date entered, known as Start date is smaller than or equal to the second date entered, known as End Date.
-
-
The String associated with
dir/
-
It is then passed into
ParseUtil#parseDirectoryString
to check if the Directory path given is existing. If the Directory path is unreal, an error is thrown to inform the user.
-
-
Please take note that:
-
If the prefix
d/
is not entered in the input, meaning that all the records will be included in the Excel sheet. -
If the prefix
dir/
is not entered in the input, meaning that the Directory Path is default as the User’s Working Directory.
-
The ArchiveCommand
has four constructors which makes use of overloading to reduce code complexity.
-
One constructor has no arguments and assigns default predicate for the
FilteredList
inModelManager
,PREDICATE_SHOW_ALL_RECORDS
which will show all items in the list and the Directory path is User’s Working Directory. -
The second constructor takes in 2
Date
arguments and assigns the predicateDateIsWithinDateIntervalPredicate
which will only show items within the date interval and the Directory path is User’s Working Directory. -
The third constructor takes in 1
Directory Path
argument and assigns the predicate asPREDICATE_SHOW_ALL_RECORDS
, which will show all items in the list and the Directory path is the entered directory path. -
The fourth constructor takes in 1
Directory Path
and 2Date
arguments and assigns the predicate asDateIsWithinDateIntervalPredicate
which will only show items within the date interval and the Directory path is the entered Directory Path.
The following sequence diagram shows how the list operation works:
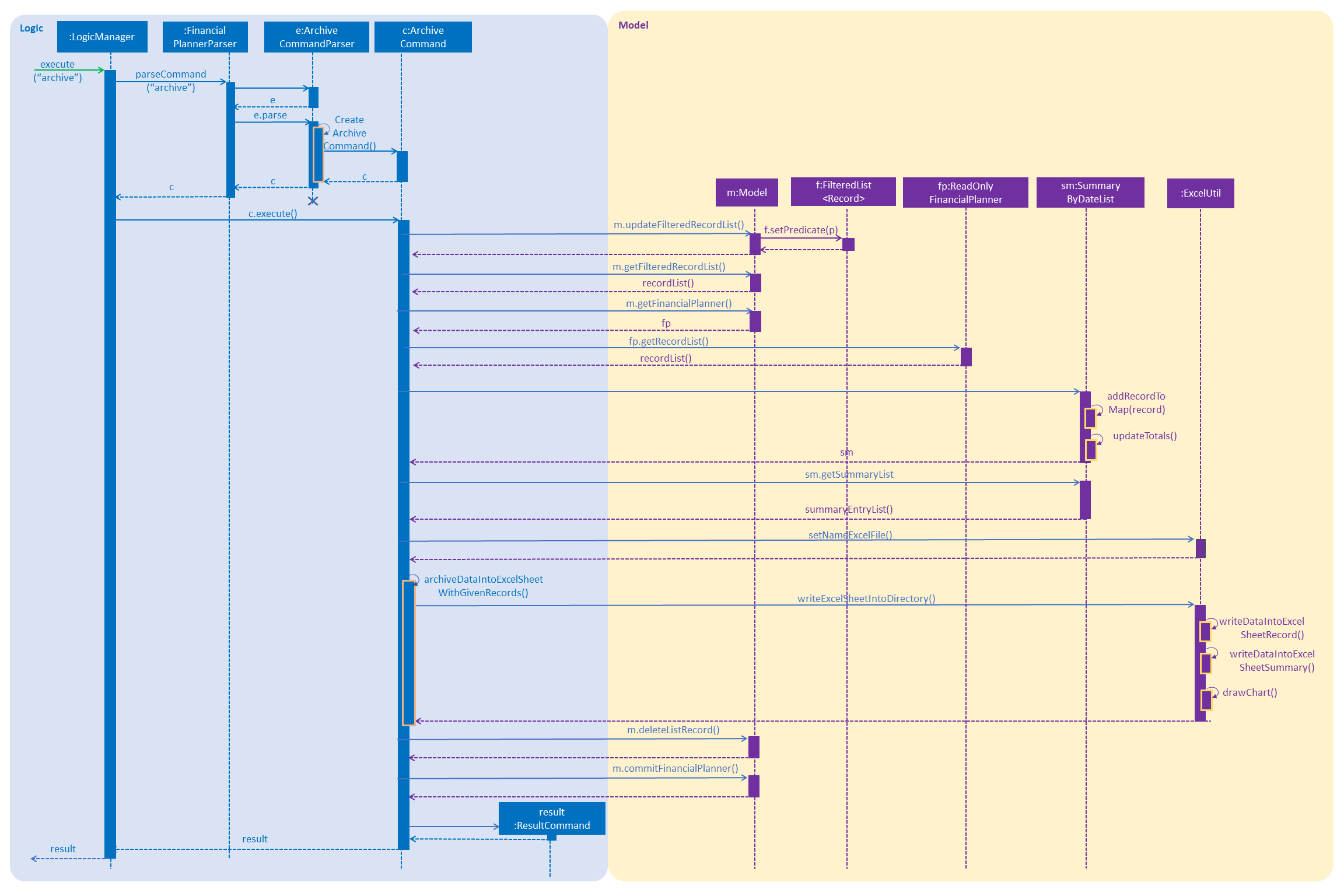
If the Excel file with the same name and stored in same Directory exists, it will be overwritten. However, it must be closed before we enter the command.
Please note that the undo
and redo
command can only affect the Savee but the not the Excel file created, meaning that when you enter undo
command after you enter the archive
command, the records archived will be added again back to the Savee but the Excel file created will not be deleted.
3.14. Draw a line chart automatically inside the Excel sheet
3.14.1. Current implementation
This feature will automatically uses the the summary data from the SUMMARY DATA
sheet in the Excel sheet after the command archive
or export_excel
is called. The feature mechanism is facilitated by ExcelUtil
, the utility created to handle all methods relating to Excel. It is the component which manages the interactions between the ExportExcelCommand or the ArchiveCommand with ExcelUtil#drawChart
.
3.15. [Proposed] Data Encryption
{Explain here how the data encryption feature will be implemented}
3.16. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.17, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.17. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
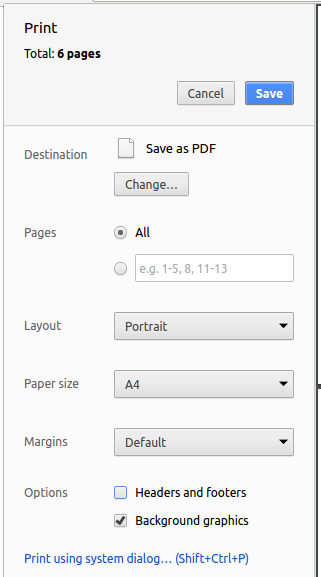
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
|
Define this attribute if the project is an official SE-EDU project. This will render the SE-EDU navigation bar at the top of the page, and add some SE-EDU-specific navigation items. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: * Official SE-EDU projects only |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
seedu.planner.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.planner.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.planner.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.planner.logic.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Financial Planner depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Suggested Programming Tasks to Get Started
Suggested path for new programmers:
-
First, add small local-impact (i.e. the impact of the change does not go beyond the component) enhancements to one component at a time. Some suggestions are given in Section A.1, “Improving each component”.
-
Next, add a feature that touches multiple components to learn how to implement an end-to-end feature across all components. Section A.2, “Creating a new command:
remark
” explains how to go about adding such a feature.
A.1. Improving each component
Each individual exercise in this section is component-based (i.e. you would not need to modify the other components to get it to work).
Logic
component
Scenario: You are in charge of logic
. During dog-fooding, your team realize that it is troublesome for the user to type the whole command in order to execute a command. Your team devise some strategies to help cut down the amount of typing necessary, and one of the suggestions was to implement aliases for the command words. Your job is to implement such aliases.
Do take a look at Section 2.3, “Logic component” before attempting to modify the Logic component.
|
-
Add a shorthand equivalent alias for each of the individual commands. For example, besides typing
clear
, the user can also typec
to remove all records in the list.
Model
component
Scenario: You are in charge of model
. One day, the logic
-in-charge approaches you for help. He wants to implement a command such that the user is able to remove a particular tag from everyone in the financial planner, but the model API does not support such a functionality at the moment. Your job is to implement an API method, so that your teammate can use your API to implement his command.
Do take a look at Section 2.4, “Model component” before attempting to modify the Model component.
|
-
Add a
removeTag(Tag)
method. The specified tag will be removed from everyone in the financial planner.
Ui
component
Scenario: You are in charge of ui
. During a beta testing session, your team is observing how the users use your financial planner application. You realize that one of the users occasionally tries to delete non-existent tags from a contact, because the tags all look the same visually, and the user got confused. Another user made a typing mistake in his command, but did not realize he had done so because the error message wasn’t prominent enough. A third user keeps scrolling down the list, because he keeps forgetting the index of the last person in the list. Your job is to implement improvements to the UI to solve all these problems.
Do take a look at Section 2.2, “UI component” before attempting to modify the UI component.
|
-
Use different colors for different tags inside record cards. For example,
friends
tags can be all in brown, andcolleagues
tags can be all in yellow.Before
After
-
Modify
NewResultAvailableEvent
such thatResultDisplay
can show a different style on error (currently it shows the same regardless of errors).Before
After
-
Modify the
StatusBarFooter
to show the total number of people in the financial plannerBefore
After
Storage
component
Scenario: You are in charge of storage
. For your next project milestone, your team plans to implement a new feature of saving the financial planner to the cloud. However, the current implementation of the application constantly saves the financial planner after the execution of each command, which is not ideal if the user is working on limited internet connection. Your team decided that the application should instead save the changes to a temporary local backup file first, and only upload to the cloud after the user closes the application. Your job is to implement a backup API for the financial planner storage.
Do take a look at Section 2.5, “Storage component” before attempting to modify the Storage component.
|
-
Add a new method
backupFinancialPlanner(ReadOnlyFinancialPlanner)
, so that the financial planner can be saved in a fixed temporary location.
A.2. Creating a new command: remark
By creating this command, you will get a chance to learn how to implement a feature end-to-end, touching all major components of the app.
Scenario: You are a software maintainer for financialplanner
, as the former developer team has moved on to new projects. The current users of your application have a list of new feature requests that they hope the software will eventually have. The most popular request is to allow adding additional comments/notes about a particular contact, by providing a flexible remark
field for each contact, rather than relying on tags alone. After designing the specification for the remark
command, you are convinced that this feature is worth implementing. Your job is to implement the remark
command.
A.2.1. Description
Edits the remark for a record specified in the INDEX
.
Format: remark INDEX r/[REMARK]
Examples:
-
remark 1 r/Likes to drink coffee.
Edits the remark for the first record toLikes to drink coffee.
-
remark 1 r/
Removes the remark for the first record.
A.2.2. Step-by-step Instructions
[Step 1] Logic: Teach the app to accept 'remark' which does nothing
Let’s start by teaching the application how to parse a remark
command. We will add the logic of remark
later.
Main:
-
Add a
RemarkCommand
that extendsCommand
. Upon execution, it should just throw anException
. -
Modify
FinancialPlannerParser
to accept aRemarkCommand
.
Tests:
-
Add
RemarkCommandTest
that tests thatexecute()
throws an Exception. -
Add new test method to
FinancialPlannerParserTest
, which tests that typing "remark" returns an instance ofRemarkCommand
.
[Step 2] Logic: Teach the app to accept 'remark' arguments
Let’s teach the application to parse arguments that our remark
command will accept. E.g. 1 r/Likes to drink coffee.
Main:
-
Modify
RemarkCommand
to take in anIndex
andString
and print those two parameters as the error message. -
Add
RemarkCommandParser
that knows how to parse two arguments, one index and one with prefix 'r/'. -
Modify
FinancialPlannerParser
to use the newly implementedRemarkCommandParser
.
Tests:
-
Modify
RemarkCommandTest
to test theRemarkCommand#equals()
method. -
Add
RemarkCommandParserTest
that tests different boundary values forRemarkCommandParser
. -
Modify
FinancialPlannerParserTest
to test that the correct command is generated according to the user input.
[Step 3] Ui: Add a placeholder for remark in RecordCard
Let’s add a placeholder on all our RecordCard
s to display a remark for each record later.
Main:
-
Add a
Label
with any random text insideRecordListCard.fxml
. -
Add FXML annotation in
RecordCard
to tie the variable to the actual label.
Tests:
-
Modify
RecordCardHandle
so that future tests can read the contents of the remark label.
[Step 4] Model: Add Remark
class
We have to properly encapsulate the remark in our Record
class. Instead of just using a String
, let’s follow the conventional class structure that the codebase already uses by adding a Remark
class.
Main:
-
Add
Remark
to model component (you can copy fromName
, remove the regex and change the names accordingly). -
Modify
RemarkCommand
to now take in aRemark
instead of aString
.
Tests:
-
Add test for
Remark
, to test theRemark#equals()
method.
[Step 5] Model: Modify Record
to support a Remark
field
Now we have the Remark
class, we need to actually use it inside Record
.
Main:
-
Add
getRemark()
inRecord
. -
You may assume that the user will not be able to use the
add
andedit
commands to modify the remarks field (i.e. the person will be created without a remark). -
Modify
SampleDataUtil
to add remarks for the sample data (delete yourfinancialPlanner.xml
so that the application will load the sample data when you launch it.)
[Step 6] Storage: Add Remark
field to XmlAdaptedRecord
class
We now have Remark
s for Record
s, but they will be gone when we exit the application. Let’s modify XmlAdaptedPerson
to include a Remark
field so that it will be saved.
Main:
-
Add a new Xml field for
Remark
.
Tests:
-
Fix
invalidAndValidPersonFinancialPlanner.xml
,typicalPersonsFinancialPlanner.xml
,validFinancialPlanner.xml
etc., such that the XML tests will not fail due to a missing<remark>
element.
[Step 6b] Test: Add withRemark() for RecordBuilder
Since Record
can now have a Remark
, we should add a helper method to RecordBuilder
, so that users are able to create remarks when building a Record
.
Tests:
-
Add a new method
withRemark()
forRecordBuilder
. This method will create a newRemark
for the record that it is currently building. -
Try and use the method on any sample
Record
inTypicalRecords
.
[Step 7] Ui: Connect Remark
field to PersonCard
Our remark label in RecordCard
is still a placeholder. Let’s bring it to life by binding it with the actual remark
field.
Main:
-
Modify
RecordCard
's constructor to bind theRemark
field to theRecord
's remark.
Tests:
-
Modify
GuiTestAssert#assertCardDisplaysPerson(…)
so that it will compare the now-functioning remark label.
[Step 8] Logic: Implement RemarkCommand#execute()
logic
We now have everything set up… but we still can’t modify the remarks. Let’s finish it up by adding in actual logic for our remark
command.
Main:
-
Replace the logic in
RemarkCommand#execute()
(that currently just throws anException
), with the actual logic to modify the remarks of a person.
Tests:
-
Update
RemarkCommandTest
to test that theexecute()
logic works.
A.2.3. Full Solution
See this PR for the step-by-step solution.
Appendix B: Product Scope
Target user profile:
-
has a need to manage his finances using other means because user is not as good at saving
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: manage income and spending faster than a typical mouse/GUI driven app
Appendix C: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
user |
be able to input my expenses and income along with a timestamp |
record how much I am spending |
|
user |
be able to delete existing entries |
remove records which I do not need |
|
user |
be able to edit existing entries |
update records with the correct information |
|
user |
be able to undo and redo |
Revert any unwanted changes |
|
user |
be able to retain my financial information when app shuts down |
Not have the app open all the time |
|
user |
be able to see the history of my financial activities |
Can keep track of my financial activities |
|
user with many records in the FinancialPlanner |
have an ordered list of records |
locate records easily |
|
user with many records in the FinancialPlanner |
find a record easily by name |
access any record I want easily |
|
user |
be able to clear history |
to reduce the clutter in the app |
|
user |
be able to create a limit for my daily final activities |
stop myself from overspending |
|
user |
be able to see a summary expenditure of each category |
see the areas where I am spending the most |
|
user |
be able to see a summary of each day or month |
see how much I am spending per day or month |
|
new user |
see usage instructions |
refer to instructions when i forget how to use the app |
|
user |
access the history within a few seconds |
do not have the time to wait for the app to update |
|
advanced user |
be able to freely edit the tags |
define my own categories |
|
user |
be able to tag my financial activities with a category |
To figure out in which part money spent on. |
|
advanced user |
export my financial activities into other forms |
log it and store it in a place different from the app |
|
user |
search based on the categories |
monitor the expenditure in a specific category |
|
user |
search based on a time period |
observe spending during different time periods, e.g holidays, school period |
|
advanced user |
plan out my future expenses |
allocate money properly, to ensure I can make my payments |
|
user |
have a visual representation of daily and monthly financial activity |
visualise the amount spend each day or month |
|
user |
have a visual representation of financial activity broken down into categories |
easily identify the areas where I am spending the most |
|
user |
record parties to make payment to |
allocate money and ensure I do not owe anyone |
|
user |
know my current monthly financial activity |
have a sense of how much I am spending currently |
|
user |
view balances in bank accounts |
keep track of my bank savings or current without having to login to accounts |
|
user |
have an intuitive user interface |
so that I don’t have to think too much when using the app |
|
user |
have a visually appealing user interface |
so that I feel happy when using the app |
|
user |
be able to access basic app configurations |
so that I can adjust the app to my liking |
|
user |
hide private details by default |
minimize chance of someone else seeing them by accident |
|
user |
a secure place to store my finance records |
keep my financial data safe |
|
user |
archive my old finance history |
look back at my finance history whenever I need it |
|
inexperienced user |
have command suggestions |
use the commands without having to remember them |
|
advanced user |
add notes to each finance record |
know where my money went |
Appendix D: Use Cases
(For all use cases below, the System is the Savee
and the Actor is the user
, unless specified otherwise)
1. Use case: Input expense for a certain activity.
MSS
-
User requests to add record into Savee.
-
Save requests for record information to be added.
-
User inputs record information.
-
System adds the record into the system.
-
System displays the record added to the user.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
Savee throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
1a. User’s input parameters are missing or invalid.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
1a. The record to be added is a duplicate of existing record in the system.
-
Savee throws duplicated record error.
-
Use case ends.
-
2. Use case: delete past finance records
MSS
-
User requests to delete record.
-
System deletes the record from the system and shows the user the result.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
Savee throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
1a. User’s input parameters are missing or invalid.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
3. Use case: update past finance records with information
MSS
-
User requests to update records and enters the parameters needed.
-
System updates the record in the system and shows the user the result.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
Savee throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
1a. User’s input parameters are missing or invalid.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
1a. The record to be added is a duplicate of existing record in the system.
-
Savee throws duplicated record error.
-
Use case ends.
-
4. Use case: See history of expenses for a certain period
MSS
-
User requests to see history within a certain date period.
-
Savee returns the history of all expenses within the period.
Use case ends.
Extensions
-
1a. User’s command is of invalid form.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
-
1a. User does not specify any arguments.
-
Savee returns all records in the Savee.
-
Use case ends.
-
-
1a. The arguments are of incorrect format
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case resumes at step 1.
-
-
2a. There is no history.
-
Savee shows a blank list.
-
Use case ends.
-
5. Use case: Delete expense entry whose date is required. [Linh Chi]
MSS
-
User requests to delete records whose date is required. User enters the date entry he/she wants to delete.
-
Savee checks whether the day required is valid.
-
Savee deletes all records whose date is required.
Use case ends.
Extensions
-
1a. User’s command is of invalid.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
-
1b. The date entered is of incorrect format.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case resumes at step 1.
-
-
1c. There is no date entered.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
6. Use case: Clear history of expenses
MSS
-
User requests to clear history of expenses.
-
System clears the history
Use case ends.
7. Use case: View breakdown of financial activity into category
MSS
-
User requests for category breakdown within a period of time.
-
System retrieves the category breakdown report.
-
System shows the report to the user in format of pie charts.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
1a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
2a. There are no records within the time range specified.
-
System shows an empty page with warning that nothing has been found.
-
Use case ends.
-
8. Use case: Search expenses based on tags [Zhi Thon]
MSS
-
User requests to search for expenses by tags
-
Savee requests for tag of expenditure
-
User enters tag desired
-
Savee displays each expenditure tagged with specified word.
Use case ends.
Extensions
-
3a User’s input tag cannot be found.
-
Savee shows no expenditure for selected category
-
9. Use case: View daily/monthly summary within a period of time
MSS
-
User requests for summary report within a period of time.
-
System retrieves the summary report.
-
System shows the report to the user in format of a table.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
1a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
2a. There are no records within the time range specified.
-
System shows an empty table.
-
Use case ends.
-
10. Use case: Usage Instructions guide
MSS
-
User requests for usage instructions
-
System returns a help window with all the instructions.
Use case ends.
11. Use case: Undo record entry
MSS
-
User requests for undo
-
Savee undo the last undoable command
Use case ends.
12. Use case: Add notes to each expense
MSS
-
User requests for adding notes and enters the notes
-
Savee adds the notes into the system and notifies the user.
Use case ends.
Extensions
* 1a. User’s input command is invalid.
Savee throws invalid command error and shows an example command input needed.
Use case ends.
-
1a. User’s input parameters are missing or invalid.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
13. Use case: Create a normal limit for a period of time or a day
MSS
-
User requests to create a normal limit for expenses different period of time or a day.
-
Savee requests for the dateStart and dateEnd of that period of time or the date of the day and the maximum amount of spending.
-
User enters the dateStart and dateEnd or the date and the amount of spending.
-
Savee records the limit in the hard drive.
-
Savee keeps tracking the spending during limit’s date period of time or that day.
-
Savee warns user when the total spend exceeds the limit amount of spending.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
3a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
3b. The dateStart is later than dateEnd.
-
System shows date error.
-
Use case ends.
-
-
3c. There is already a limit for the time period or the day.
-
System shows redundant limit error.
-
Use case ends.
-
14. Use case: Edit an existing normal limit
MSS
-
User requests to edit an existing normal limit.
-
Savee requests for the new maximum amount of spending for the period of time or the day.
-
User enters the dateStart and dateEnd or the date and the new amount of spending.
-
Savee updates the new limit in the hard drive.
-
Savee keeps tracking the spending during the limit’s date period of time or that day.
-
Savee warns user when the total spend exceeds the limit amount of spending.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
3a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
3b. The dateStart is later than dateEnd.
-
System shows date error.
-
Use case ends.
-
-
3c. There is no existing limit for the time period or the day.
-
System shows no existing limit error.
-
Use case ends.
-
-
3d. The edit limit is exactly the same as the original one.
-
System shows same limit error.
-
Use case ends.
-
15. Use case: Delete an existing limit
MSS
-
User requests to delete an existing limit.
-
Savee requests for the date period of the limit to be deleted.
-
User enters the dateStart and dateEnd or the date.
-
Savee deletes the limit in the hard drive.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
3a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
3b. The dateStart is later than dateEnd.
-
System shows date error.
-
Use case ends.
-
-
3c. There is no existing limit for the time period or the day.
-
System shows no existing limit error.
-
Use case ends.
-
16. Use case: Add a monthly limit
MSS
-
User requests to add the monthly limit.
-
Savee requests the monthly maximum amount of spending.
-
User enters the maximum amount of spending.
-
Savee adds the monthly limit.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
3a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
3b. There is already a monthly limit.
-
System shows existing monthly limit error.
-
Use case ends.
-
17. Use case: Edit the monthly limit
MSS
-
User requests to edit the monthly limit.
-
Savee requests the new monthly maximum amount of spending.
-
User enters the new maximum amount of spending.
-
Savee updates the monthly limit.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
3a. User’s input parameters are missing or invalid.
-
System throws invalid command error and notifies user of the correct format.
-
Use case ends.
-
-
3b. There is no monthly limit.
-
System shows no monthly limit error.
-
Use case ends.
-
18. Use case: Delete the monthly limit
MSS
-
User requests to delete the monthly limit.
-
Savee updates the monthly limit.
Use case ends.
Extensions
-
1a. User’s input command is invalid.
-
System throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
2a. There is no monthly limit.
-
System shows no monthly limit error.
-
Use case ends.
-
19. Use case: Check all the existing limits
MSS
-
User requests to check all the existing limits.
-
Savee displays all the existing limits.
Use case ends.
20. Use case: Export record data from Savee to Excel file [Linh Chi]
MSS
-
User requests to export the data from Savee to Excel file.
-
Savee exports the records data in Excel file.
-
Savee informs the user the directory where the Excel file is saved.
Extensions
-
1a. User’s command is of invalid form.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
-
1b. The parameters entered is of incorrect format.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case resumes at step 1.
-
-
1c. The directory path parameters entered is non-existent.
-
Savee throws invalid command error and notifies user of the user non-existent directory path.
-
Use case resumes at step 1.
-
-
1d. The user enters the parameters but does not enter the prefix.
-
Savee throws invalid command error.
-
Use case resumes at the step 1.
-
-
1e. The user enters the prefix but does not enter the parameters.
-
Savee throws invalid command error.
-
Use case resumes at the step 1.
-
-
1f. The user enters more than 3 dates.
-
Savee throws invalid command error and notifies user the maximum number of dates enter is 2.
-
Use case resumes at step 1.
-
-
1g. The user enters exact 2 dates and the first date is later than the second date.
-
Savee throws invalid command error and notifies user the first data should be earlier or equal to the second date.
-
Use case resumes at step 1.
-
21. Use case: Import the Excel file into Financial Planner. [Linh Chi]
MSS
-
User requests to import the record data from Excel file to Financial Planner.
-
Savee reads the Excel file and transfer all the data in the Excel file into Record object and add them into current Savee.
Extensions
-
1a. User’s command is of invalid form.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
-
1b. User input wrong file path that the Excel file is stored.
-
Savee throws invalid command error and notifies the user the directory path is not existent.
-
Use case resumes at step 1.
-
-
1c. User input the parameter but does not enter the prefix.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
22. Use case: Archive the records data into Excel file. [Linh Chi]
MSS
-
User requests to archive the record from Savee to Excel file.
-
Savee archives the records data in Excel file.
-
Savee informs the user the directory where the Excel file is saved.
Extensions
-
1a. User’s command is of invalid form.
-
Savee throws invalid command error.
-
Use case resumes at step 1.
-
-
1b. The parameters entered is of incorrect format.
-
Savee throws invalid command error and notifies user of the correct format.
-
Use case resumes at step 1.
-
-
1c. The directory path parameters entered is non-existent.
-
Savee throws invalid command error and notifies user of the user non-existent directory path.
-
Use case resumes at step 1.
-
-
1d. The user enters the parameters but does not enter the prefix.
-
Savee throws invalid command error.
-
Use case resumes at the step 1.
-
-
1e. The user enters the prefix but does not enter the parameters.
-
Savee throws invalid command error.
-
Use case resumes at the step 1.
-
-
1f. The user enters more than 3 dates.
-
Savee throws invalid command error and notifies user the maximum number of dates enter is 2.
-
Use case resumes at step 1.
-
-
1g. The user enters exact 2 dates and the first date is later than the second date.
-
Savee throws invalid command error and notifies user the first data should be earlier or equal to the second date.
-
Use case resumes at step 1.
-
23. Use case: See monthly financial activity
MSS
-
User requests to see monthly financial activity.
-
Savee returns a report on monthly financial activity.
24. Use case: Freely edit expenses tags
MSS
-
User requests to edit expenses tags.
-
Savee requests for date of entry to edit tags
-
User enters date to edit entry for
-
Savee shows list of entries on specified date
-
User enters entry to edit.
-
Savee shows current tags of selected entry.
-
User enters tag to remove
-
Savee removes tag entered.
Extensions
-
3a User inputs invalid date
-
Savee throws invalid date error.
-
Use case resumes at step 2.
-
-
3b Date input has no entries to edit
-
Savee throws no entry error.
-
Use case resumes at step 2.
-
-
5a User’s input is not a valid entry.
-
Savee throws invalid data error and resumes at step 4.
-
-
5a User’s input entry has no tags.
-
Savee displays ‘No tags’ and continues to step 7.
-
-
7a User’s input tag does not exist
-
Savee adds tag to entry.
-
25. View bank balance
MSS
-
User requests to view bank balance.
-
Savee asks for authentication.
-
User provides authentication.
-
Savee verifies the authentication and shows the bank balance.
-
1a. User’s input command is invalid.
-
Savee throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
3a. User’s authentication is invalid.
-
Savee returns error and asks for authentication again.
-
Use case resumes at 2.
-
-
26. Adjust configurations
MSS
-
User requests to change application configurations.
-
Savee returns the configuration page.
-
User changes configuration.
-
Savee confirms the configuration and applies it to the system.
-
1a. User’s input command is invalid.
-
Savee throws invalid command error and shows an example command input needed.
-
Use case ends.
-
-
Appendix E: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to hold up to 1000 records without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Should be able to retain data in the event of app exiting or crashing.
-
The data is not expected to be secure.
-
The application can function as per normal in the absence of network.
-
For best view, the optimal resolution is 1080p (1920 x 1080).
Appendix F: Glossary
Appendix G: Product Survey
Product Name
Author: …
Pros:
-
…
-
…
Cons:
-
…
-
…
Appendix H: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
H.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample records. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
H.2. Listing by date
-
Listing with no argument
-
Test case: Enter
list
-
Expected: System would list down all the records along with a result saying that it has listed all records.
-
-
Listing with arguments but no prefix
-
Test case: Enter
list abcd
-
Expected: System will throw error saying invalid command and show the correct format
-
-
Listing with arguments but wrong prefix
-
Test case: Enter
list m/1-1-2018 12-12-2018
-
Expected: System will throw error saying invalid command and show the correct format
-
-
Listing with valid prefix but invalid arguments
-
Test case: Enter
list d/1-1-201
-
Expected: System will throw error saying invalid command and show the correct format
-
-
Listing with valid prefix but 1 date
-
Test case: Enter
list d/1-1-2018
-
Expected: System will list only records of that date and show a command result stating the date that the records are listed by
-
-
Listing with valid prefix and 2nd date earlier than 1st date
-
Test case: Enter
list d/1-1-2018 12-12-2017
-
Expected: System will throw error saying invalid command and show the correct format
-
-
Listing with valid prefix and 1st date earlier than 2nd date
-
Test case: Enter
list d/1-1-2018 12-12-2018
-
Expected: System will list all records equal to or between the 2 dates
-
H.3. Sorting records
-
Sorting with no argument
Test case: Entersort
Expected: System will throw error saying invalid command and show the correct format -
Sorting with invalid argument
Test case: Entersort invalidargument
Expected: System will throw error saying invalid command and show the correct format -
Sorting with valid and invalid argument
Test case: Entersort money haha
Expected: System will throw error saying invalid command and show the correct format -
Sorting with name only
Test case: Entersort
Expected: System will sort all records by date in ascending order. -
Sorting with date only
Test case: Entersort date
Expected: System will sort all records by date in ascending order. -
Sorting with money only
Test case: Entersort money
Expected: System will sort all records by money in ascending order. -
Sorting with ascending order only
Test case: Entersort asc
Expected: System will sort all records by name in ascending order. -
Sorting with descending order only
Test case: Entersort desc
Expected: System will sort all records by name in ascending order. -
Sorting with no argument
Test case: Entersort money desc
Expected: System will sort all records by money in descending order.
H.4. Finding Records by Tag
-
Sorting with no argument
Test case: Enterfindtag
Expected: System will throw error saying invalid command and show the correct format -
Sorting with single argument
.est case: Enterfindtag anystring
Expected: System will display all records with the taganystring
and display message showing how many records were found and the tag searched. -
Sorting with multiple arguments
Test case: Enterfindtag anystring haha funny
Expected: System will display all records with the taganystring
haha
funny
and display message showing how many records were found and the tags searched.
H.5. Viewing summary
-
Entering
summary
command without modes-
Test case: Enter
summary abcd
-
Expected: System would throw an error saying invalid command and provide the error message for the generic summary command
-
-
Entering
summary
command with modes but no arguments-
Test case: Enter
summary date
orsummary month
orsummary category
-
Expected:
-
System would throw an error saying invalid command.
-
System will provide a different error message depending on the mode and provide more information about the format of the command in that specific mode.
-
for e.g.
summary date
will give you an error message specific to viewing summary by date andsummary month
will give you and error message specific to viewing summary by month.
-
-
Entering
summary
command with modes and invalid prefixes or no prefix-
Test case: Enter
summary date m/
orsummary month m/
orsummary category m/
-
Expected: System would throw error saying invalid command and provide the error message for the generic summary command
-
-
Entering
summary
command with modes and date prefix but only 1 argument-
Test case: Enter
summary date d/1-1-2018
orsummary month d/1-1
orsummary category d/1-1-1
-
Expected:
-
System would throw an error saying invalid command.
-
System will provide a different error message depending on the mode and provide more information about the format of the command in that specific mode.
-
for e.g.
summary date
will give you an error message specific to viewing summary by date andsummary month
will give you and error message specific to viewing summary by month.
-
-
Entering
summary
command with modes and 2 arguments with either invalid-
Test case: Enter
summary date d/1—1 1—1
orsummary month d/1---1 1—1
or summary category d/apr-2018 1—1` -
Expected:
-
System would throw error regarding the constraints of dates/months depending on the mode. If mode is
date
orcategory
, the error message is about the date. If mode ismonth
, the error message will be about the month. -
The error message should give reasons why the argument is invalid.
-
-
View summary of range with no records inside
-
Prerequisite: Choose 2 dates/months such that no records are inside that range and select a record in the list.
-
Test case: Execute the summary command.
-
Expected: The table should be empty with just an entry saying total is 0 for all the money columns and record should be unselected.
-
-
View summary of range with some records inside
-
Prerequisite: Choose 2 dates/months such that records have these characteristics and select a record in the list.
-
Test case: Execute the summary command.
-
Expected:
-
Record should be unselected.
-
The table would be filled and the total is reflective of all the entries within the table.
-
If the table is too long, the scrollbars should allow you to view all the entries.
-
H.6. Viewing statistics about category breakdown
-
Entering
stats
command without arguments and prefixes-
Test case: Enter
stats
-
Expected: System would throw an error saying invalid command and show the format the command should have been in.
-
-
Entering
stats
command with valid prefixes but no arguments-
Test case: Enter
stats d/
-
Expected: System would throw an error saying invalid command and show the format the command should have been in.
-
-
Entering
stats
command with invalid prefixes-
Test case: Enter
stats m/
-
Expected: System would throw an error saying invalid command and show the format the command should have been in.
-
-
Entering
stats
command with valid prefixes but invalid arguments-
Test case: Enter
stats d/1—1
-
Expected: System would throw an error regarding the dates entered and give reasons for why the argument is invalid.
-
-
View statistics of a range with no records inside
-
Prerequisite: Choose 2 dates such that no records are inside that range and select a random record in the list.
-
Test case: Execute the stats command.
-
Expected:
-
The record would be unselected.
-
The 2 tabs should still be created but have a message that tells user that nothing has been found.
-
-
View statistics of a range with some records inside but all income/expense
-
Prerequisites: Choose 2 dates such that the records have these characteristics and select a random record in the list.
-
Test case: Execute the stats command.
-
Expected:
-
The record would be unselected.
-
The 2 tabs should still be created but 1 of the tab would have a message telling user that nothing has been found
-
-
View statistics of a range with records with income and records with expense
-
Prerequisites: Choose 2 dates such that the records have these characteristics and select a random record in the list
-
Test case: Execute the stats command.
-
Expected:
-
The record would be unselected.
-
The 2 tabs should be created with pie charts.
-
-
View statistics of a range with too many tags
-
Prerequisites: Choose 2 dates such that the records have these characteristics and select a random record in the list
-
Test case: Execute the stats command
-
Expected:
-
The record would be unselected.
-
The 2 tabs should be created with pie charts. The legends should have scrollbars attached if there are too many tags. These scrollbars should allow you to navigate to the hidden tags within the legend.
-
H.7. Viewing current month’s breakdown
-
Navigating to welcome screen from anywhere using keyboard
-
Prerequisites: Click on any record in the list. Panel should change to display details of the record along with an image.
-
Test case: Press
HOME
on the keyboard -
Expected: Panel should show the welcome screen.
-
-
Navigating to welcome screen from anywhere automatically
-
Prerequisites: Same as before. Just need to change the panel to see the effect.
-
Test case: Add any record with date in current month/ Delete any record with date in current month/ Edit any record to a date in current month/ Clear
-
Expected: Panel should show the welcome screen.
-
H.8. Adding a normal limit
-
Adding a normal limit to the Financial Planner without the same time period limits inside.
-
Prerequisites: Check all the existing limit using the
checklimit
command. There is no limit with date period "11-11-2018 — 17-11-2018" and "12-11-2018". -
Test case:
addlimit d/11-11-2018 17-11-2018 m/100
Expected: The information shows that the new limit has been added followed by detailed information about the newly added limit. -
Test case:
addlimit d/12-11-2018 m/200
Expected: The information shows that the new limit has been added followed by detailed information about the newly added limit.
-
-
Trying to add a normal limit to the Financial Planner with command errors.
-
Test case:
addlimit d/17-11-2018 11-11-2018 m/200
Expected: No limit will be added. Error message about the dateStart must be earlier than dateEnd will be displayed. -
Test case:
addlimit d/11-11-2018 17-11-2018 m/+200
Expected: No limit will be added. Error message about wrong type of limit MoneyFlow will be displayed. -
Test case:
addlimit d/11-11-2018 17-11-2018
Expected: No limit will be added. Error message about wrong addlimit command format will be displayed. -
Test case:
addlimit 11-11-2018 17-11-2018 m/100
Expected: No limit will be added. Error message about wrong addlimit command format will be displayed.
-
-
Trying to add a normal limit to the Financial Planner with the same time period limits inside.
-
Prerequisites: Check all the existing limit using the
checklimit
command. There are existing limits with date period "11-11-2018 — 17-11-2018" and "12-11-2018". -
Test case:
addlimit d/11-11-2018 17-11-2018 m/100
Expected: No limit will be added. Error message about already existing limit will be displayed. -
Test case:
addlimit d/12-11-2018 m/100
Expected: No limit will be added. Error message about already existing limit will be displayed. -
Other incorrect addlimit commands to try:
addlimit asd `, `addlimit x
(where x is an integer),addlimit x/12-11-2018 m/asd
Expected: No limit will be added, related error information will be displayed.
-
H.9. Editing an existing normal limit
-
Editing an existing normal limit in the Financial Planner
-
Prerequisites: Check all the existing limit using the
checklimit
command. There are limits with date period "11-11-2018 — 17-11-2018" with the limit MoneyFlow isn’t "100" and "12-11-2018" with the limit MoneyFlow isn’t "200". -
Test case:
editlimit d/11-11-2018 17-11-2018 m/100
Expected: The information shows that the limit has been edited followed by detailed information about both the original limit and the new limit. -
Test case:
editlimit d/12-11-2018 m/200
Expected: The information shows that the limit has been edited followed by detailed information about both the original limit and the new limit.
-
-
Trying to edit a normal limit in the Financial Planner with command errors.
-
Test case:
editlimit d/17-11-2018 11-11-2018 m/200
Expected: No limit will be edited. Error message about the dateStart must be earlier than dateEnd will be displayed. -
Test case:
editlimit d/11-11-2018 17-11-2018 m/+200
Expected: No limit will be edited. Error message about wrong type of limit MoneyFlow will be displayed. -
Test case:
editlimit d/11-11-2018 17-11-2018
Expected: No limit will be edited. Error message about wrong editlimit command format will be displayed. -
Test case:
editlimit 11-11-2018 17-11-2018 m/200
Expected: No limit will be edited. Error message about wrong editlimit command format will be displayed.
-
-
Trying to edit a normal limit in the Financial Planner with wrong command format.
-
Prerequisites: Check all the existing limit using the
checklimit
command. There is no existing limit with date period "11-11-2018 — 17-11-2018" and "12-11-2018". -
Test case:
editlimit d/11-11-2018 17-11-2018 m/100
Expected: No limit will be edited. Error message about no existing limit will be displayed. -
Test case:
editlimit d/12-11-2018 m/100
Expected: No limit will be edited. Error message about no existing limit will be displayed.
-
-
Trying to edit a normal limit in the Financial Planner with the same limit inside.
-
Prerequisites: Check all the existing limit using the
checklimit
command. There is no existing limit with date period "11-11-2018 — 17-11-2018" with the limit MoneyFlow isn’t "100". -
Test case:
editlimit d/11-11-2018 17-11-2018 m/100
Expected: No limit will be edited. Error message about same limit will be displayed. -
Other incorrect editlimit commands to try:
editlimit asd `, `editlimit x
(where x is an integer),editlimit x/12-11-2018 m/asd
Expected: No limit will be edited, related error information will be displayed.
-
H.10. Deleting an existing normal limit
-
Deleting an existing normal limit in the Financial Planner
-
Prerequisites: Check all the existing limit using the
checklimit
command. There are limits with date period "11-11-2018 — 17-11-2018" and "12-11-2018". -
Test case:
deletelimit d/11-11-2018 17-11-2018
Expected: The information shows that the limit has been deleted. -
Test case:
deletelimit d/12-11-2018
Expected: The information shows that the limit has been deleted.
-
-
Trying to delete a normal limit in the Financial Planner with command format error.
-
Test case:
deletelimit d/17-11-2018 11-11-2018
Expected: No limit will be deleted. Error message about the dateStart must be earlier than dateEnd will be displayed. -
Test case:
deletelimit 11-11-2018 17-11-2018 m/100
Expected: No limit will be deleted. Error message about wrong deletelimit command format will be displayed. -
Prerequisites: Check all the existing limit using the
checklimit
command. There is no existing limit with date period "11-11-2018 — 17-11-2018" and "12-11-2018". -
Test case:
deletelimit d/11-11-2018 17-11-2018
Expected: No limit will be deleted. Error message about no existing limit will be displayed. -
Test case:
deletelimit d/12-11-2018
Expected: No limit will be deleted. Error message about no existing limit will be displayed. -
Other incorrect delete commands to try:
deletelimit asd `, `deletelimit x
(where x is an integer),deletelimit x/12-11-2018 m/asd
Expected: No limit will be deleted, related error information will be displayed.
-
H.11. Adding a monthly limit
-
Adding a monthly limit to the Financial Planner
-
Prerequisites: Check all the existing limit using the
checklimit
command. There is no monthly limit in the FinancialPlanner. -
Test case:
addmonthlylimit m/500
Expected: The monthly limit will be added, detailed monthly limit’s information will be displayed.
-
-
Trying to add a monthly limit to the Financial Planner with command format error.
-
Test case:
addmonthlylimit 500
Expected: No limit will be added. Error message about wrong addmonthlylimit command format will be displayed. -
Prerequisites: Check all the existing limit using the
checklimit
command. There is already an existing monthly limit. -
Test case:
addmonthlylimit m/200
Expected: No limit will be added. Error message about existing monthly limit will be displayed. -
Other incorrect addmonthlylimit commands to try:
addmonthlylimit asd `, `addmonthlylimit m/asd
Expected: The monthly limit will not be added, related error information will be displayed. === Editing the monthly limit
-
-
Editing the monthly limit existing the Financial Planner
-
Prerequisites: Check all the existing limit using the
checklimit
command. There is already a monthly limit in the FinancialPlanner. -
Test case:
editmonthlylimit m/500
Expected: The monthly limit will be edited, detailed information for both original monthly limit and new monthly limit will be displayed.
-
-
Trying to edit the monthly limit in the Financial Planner with command format error.
-
Test case:
editmonthlylimit 500
Expected: No limit will be edited. Error message about wrong editmonthlylimit command format will be displayed. -
Prerequisites: Check all the existing limit using the
checklimit
command. There is no existing monthly limit. -
Test case:
editmonthlylimit m/200
Expected: No limit will be edited. Error message about no monthly limit will be displayed. -
Other incorrect editmonthlylimit commands to try:
editmonthlylimit asd `, `editmonthlylimit m/asd
Expected: The monthly limit will not be edited, related error information will be displayed.
-
H.12. Deleting the monthly limit
-
Deleting the monthly limit existing the Financial Planner
-
Prerequisites: Check all the existing limit using the
checklimit
command. There is already a monthly limit in the FinancialPlanner. -
Test case:
deletemonthlylimit
Expected: The monthly limit will be deleted. -
Prerequisites: Check all the existing limit using the
checklimit
command. There is no existing monthly limit. -
Test case:
deletemonthlylimit
Expected: The monthly limit will not be deleted, no monthly limit error message will be displayed.
-
H.13. Checking all the limits
-
Checking all limits
-
Prerequisites: There are limits inside the LimitList.
-
Test case:
checklimit
Expected: All the detailed limits' information will be displayed. -
Prerequisites: No limits inside the limit list.
-
Test case:
checklimit
Expected: The limits' detailed information will not be displayed, no limit in the list error message will be displayed. === Deleting a person
-
-
Deleting a records while all records are listed
-
Prerequisites: List all records using the
list
command. Multiple persons in the list. -
Test case:
delete 1
Expected: First record is deleted from the list. Details of the deleted record shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No record is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size)
Expected: Similar to previous.
-
H.14. Deleting all records whose date is required.
-
Deleting all records whose date is required when all records are listed.
-
Prerequisites: List all records using the
list
command. Multiple records in the list. -
Test case:
deletedate 31-3-1999
Expected: If there are any record whose date is 31-3-1999 or 31-03-1999, these records will be deleted. The message of successful deleting records will be shown. Timestamp in the status bar is updated.
If there is no records whose date is 31-3-1999 or 31-03-1999, the message of no record existing will be shown. Status bar remains the same. -
Incorrect delete commands to try:
delete 30-2-2018
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same. -
Incorrect delete commands to try:
delete 30-2A-2018
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same. -
Incorrect delete commands to try:
deletedate
+ Expected: Invalid command entered. Error indicating invalid command format is shown. Status bar remains the same. -
Incorrect delete commands to try:
deletedate x
(where x is invalid date, meaning that date have alphabetical character or non-existent date)
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same.
-
H.15. Archiving records data from Savee to Excel file and store it in a specific directory.
-
Archiving records whose date is required when all records are listed.
-
Prerequisites: List all records using the
list
command. Multiple records in the list. -
Test case:
archive
Expected: All records will be shown. The message of successful archiving records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated. -
Test case:
archive d/31-3-1999
Expected: If there are any record whose date is 31-3-1999 or 31-03-1999, these records will be shown. The message of successful archiving records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is 31-3-1999 or 31-03-1999, the message of no record archived will be shown. Status bar remains the same. -
Test case:
archive d/31-3-1999 31-03-2019
Expected: If there are any record whose date is within period from 31-3-1999 to 31-03-2019, these records will be shown. The message of successful archiving records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is is within period from 31-3-1999 to 31-03-2019, the message of no record archived will be shown. Status bar remains the same. -
Test case:
archive dir/C:\
Expected: All records will be shown. The message of successful archiving records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated. -
Test case:
archive dir/C:\ d/31-3-1999
Expected: If there are any record whose date is 31-3-1999 or 31-03-1999, these records will be shown. The message of successful archiving records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is 31-3-1999 or 31-03-1999, the message of no record archived will be shown. Status bar remains the same. -
Test case:
archive dir/C:\ d/31-3-1999 31-03-2019
Expected: If there are any record whose date is within period from 31-3-1999 to 31-03-2019, these records will be shown. The message of successful archiving records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is is within period from 31-3-1999 to 31-03-2019, the message of no record archived will be shown. Status bar remains the same. -
Incorrect archive commands to try:
archive d/30-2-2018
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same. -
Incorrect archive commands to try:
archive d/30-2A-2018
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same. -
Incorrect archive commands to try:
archive 31-3-1999
+ Expected: Invalid command entered. Error indicating invalid command format is shown. Status bar remains the same. -
Incorrect archive commands to try:
archive C:\
+ Expected: Invalid command entered. Error indicating invalid command format is shown. Status bar remains the same.
-
H.16. Import all record data from Excel file to Savee
-
Import record data from Excel file into Savee.
-
Prerequisites:
archive dir/C:\
-
Test case:
import dir/C:\ n/Financial_Planner_ALL.xlsx
Expected: All record data from the Excel file are shown in Savee. -
Test case:
import dir/C:\ n/Financial_Planner_ALL
Expected: All record data from the Excel file are shown in Savee. -
Test case:
import dir/C:\Financial_Planner_ALL.xlsx
Expected: All record data from the Excel file are shown in Savee. -
Incorrect import commands to try:
import
Expected: Invalid date entered. Error indicating invalid command format. Status bar remains the same.
-
H.17. Exporting records data from Savee to Excel file and store it in a specific directory.
-
Exporting records whose date is required when all records are listed.
-
Prerequisites: List all records using the
list
command. Multiple records in the list. -
Test case:
exportexcel
Expected: All records will be shown. The message of successful exporting records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated. -
Test case:
exportexcel d/31-3-1999
Expected: If there are any record whose date is 31-3-1999 or 31-03-1999, these records will be shown. The message of successful exporting records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is 31-3-1999 or 31-03-1999, the message of no record exported will be shown. Status bar remains the same. -
Test case:
exportexcel d/31-3-1999 31-03-2019
Expected: If there are any record whose date is within period from 31-3-1999 to 31-03-2019, these records will be shown. The message of successful exporting records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is is within period from 31-3-1999 to 31-03-2019, the message of no record exported will be shown. Status bar remains the same. -
Test case:
exportexcel dir/C:\
Expected: All records will be shown. The message of successful exporting records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated. -
Test case:
exportexcel dir/C:\ d/31-3-1999
Expected: If there are any record whose date is 31-3-1999 or 31-03-1999, these records will be shown. The message of successful exporting records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is 31-3-1999 or 31-03-1999, the message of no record exported will be shown. Status bar remains the same. -
Test case:
exportexcel dir/C:\ d/31-3-1999 31-03-2019
Expected: If there are any record whose date is within period from 31-3-1999 to 31-03-2019, these records will be shown. The message of successful exporting records will be shown together with the Excel file name and the directory path it will be shown. Timestamp in the status bar is updated.
If there is no records whose date is is within period from 31-3-1999 to 31-03-2019, the message of no record exported will be shown. Status bar remains the same. -
Incorrect export commands to try:
exportexcel d/30-2-2018
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same. -
Incorrect export commands to try:
exportexcel d/30-2A-2018
Expected: Invalid date entered. Error indicating invalid command format and notifies user that the date entered is of invalid format. Status bar remains the same. -
Incorrect export commands to try:
exportexcel 31-3-1999
+ Expected: Invalid command entered. Error indicating invalid command format is shown. Status bar remains the same. -
Incorrect export commands to try:
exportexcel C:\
+ Expected: Invalid command entered. Error indicating invalid command format is shown. Status bar remains the same.
-
H.18. Auto Complete Feature
-
Typing part of a command word
Test case: Enterad
.
Expected: Suggestions pop up with the words "add", "addlimit", "addmonthlylimit". -
Typing a complete command word
Test case: Enteradd
.
Expected: No Suggestions appear. -
Typing a complete command word with extra text
Test case: Enteraddm
.
Expected: Suggestions pop up with the word "addmonthlylimit" only. -
Typing name arguments for add command word Test case: Enter
add n/s
.
Expected: Suggestions pop up with the words that begin with "s", only if there are record names that have words that begin with 's'. -
Typing name arguments for add command word Test case: Enter
add n/Buying it
.
Expected: Suggestions pop up with the words that begin with "it", only if there are record names that have words that begin with 'it'. -
Typing date arguments for add command word Test case: Enter
add d/1
.
Expected: Suggestions pop up with the dates that begin with "1", only if there are record dates that have words that begin with '1'. Does not show dates that begin with '01'. -
Typing date arguments for add command word Test case: Enter
add d/t
.
Expected: Suggestions pop up with the word "today" only. -
Typing moneyflow arguments for add command word Test case: Enter
add m/1
.
Expected: No suggestions popup no matter what records are in the data. No suggestion expected for moneyflow at all. -
Typing tag arguments for add command word Test case: Enter
add t/f
.
Expected: Suggestions pop up with the words that begin with "f", only if there are record tags that have words that begin with 'f', such as food and family, which are some default words for tags. -
Typing name arguments for add command word with a 'n/' prefix Test case: Enter
add n/Buying m/-50 it
.
Expected: No suggestions pop up for 'it' no matter what. No suggestions are expected for this invalid input. -
Typing date arguments for list command word Test case: Enter
list t
.
Expected: no suggestions pop up as prefix is required. -
Typing date arguments for list command word with a 'd/' prefix Test case: Enter
list d/t
.
Expected: Suggestions pop up with the word "today" only. -
Typing date arguments for list command word after a date with 'd/' prefix Test case: Enter
list d/ytd t
.
Expected: Suggestions pop up with the word "today" only. -
Typing date arguments for list command word after a date with 'd/' prefix Test case: Enter
list d/ytd t
.
Expected: Suggestions pop up with the word "today" only. -
Typing arguments for sort command word Test case: Enter
sort d
.
Expected: Suggestions pop up with the words "date" and "desc" only. Any category or order can be suggested. -
Typing arguments for sort command word with a category specified Test case: Enter
sort money d
.
Expected: Suggestions pop up with the word "desc" only. Only order can be suggested. -
Typing arguments for sort command word with a order specified Test case: Enter
sort asc m
.
Expected: Suggestions pop up with the words "money" and "moneyflow" only. Only category can be suggested. -
Typing arguments for sort command word with a order specified Test case: Enter
sort asc m
.
Expected: Suggestions pop up with the words "money" and "moneyflow" only. Only category can be suggested. -
Typing date arguments for addlimit command word Test case: Enter
addlimit d/t
.
Expected: Suggestions pop up with the word "today" only. -
Typing secondary date arguments for addlimit command word Test case: Enter
addlimit d/ytd t
.
Expected: Suggestions pop up with the word "today" only. -
Typing date arguments for addlimit command word with money included Test case: Enter
addlimit m/999 d/ytd t
.
Expected: Suggestions pop up with the word "today" only. -
Typing date arguments for addlimit command word with money included Test case: Enter
addlimit d/ytd t m/999
. Move the caret in the command box back to right after the 't'. Expected: Caret will be stopped right after 't' and suggestions pop up with the word "today" only.
H.19. Saving data
-
Dealing with missing/corrupted data files
-
Prerequisites: Have a data file ready. Go to the application and add a record. A data xml file should be created.
-
Test case: Go to the file and remove 1 of the field tags. It should look like "<name></name>" where name is the name of the field. Then, open the app.
-
Expected: The app should initialise with an empty record list.
-
Test case: Delete the data file
-
Expected: The app should initialise with a sample record list.
-